discworld coordinates
Ben Cordes
Shared posts
Algorithms interviews: theory vs. practice
When I ask people at trendy big tech companies why algorithms quizzes are mandatory, the most common answer I get is something like "we have so much scale, we can't afford to have someone accidentally write an O(n^2)
algorithm and bring the site down"1. One thing I find funny about this is, even though a decent fraction of the value I've provided for companies has been solving phone-screen level algorithms problems on the job, I can't pass algorithms interviews! When I say that, people often think I mean that I fail half my interviews or something. It's more than half.
When I wrote a draft blog post of my interview experiences, draft readers panned it as too boring and repetitive because I'd failed too many interviews. I should summarize my failures as a table because no one's going to want to read a 10k word blog post that's just a series of failures, they said (which is good advice; I'm working on a version with a table). I’ve done maybe 40-ish "real" software interviews and passed maybe one or two of them (arguably zero)2.
Let's look at a few examples to make it clear what I mean by "phone-screen level algorithms problem", above.
At one big company I worked for, a team wrote a core library that implemented a resizable array for its own purposes. On each resize that overflowed the array's backing store, the implementation added a constant number of elements and then copied the old array to the newly allocated, slightly larger, array. This is a classic example of how not to implement a resizable array since it results in linear time resizing instead of amortized constant time resizing. It's such a classic example that it's often used as the canonical example when demonstrating amortized analysis.
For people who aren't used to big tech company phone screens, typical phone screens that I've received are one of:
- an "easy" coding/algorithms question, maybe with a "very easy" warm-up question in front.
- a series of "very easy" coding/algorithms questions,
- a bunch of trivia (rare for generalist roles, but not uncommon for low-level or performance-related roles)
This array implementation problem is considered to be so easy that it falls into the "very easy" category and is either a warm-up for the "real" phone screen question or is bundled up with a bunch of similarly easy questions. And yet, this resizable array was responsible for roughly 1% of all GC pressure across all JVM code at the company (it was the second largest source of allocations across all code) as well as a significant fraction of CPU. Luckily, the resizable array implementation wasn't used as a generic resizable array and it was only instantiated by a semi-special-purpose wrapper, which is what allowed this to "only" be responsible for 1% of all GC pressure at the company. If asked as an interview question, it's overwhelmingly likely that most members of the team would've implemented this correctly in an interview. My fixing this made my employer more money annually than I've made in my life.
That was the second largest source of allocations, the number one largest source was converting a pair of long
values to byte arrays in the same core library. It appears that this was done because someone wrote or copy pasted a hash function that took a byte array as input, then modified it to take two inputs by taking two byte arrays and operating on them in sequence, which left the hash function interface as (byte[], byte[])
. In order to call this function on two longs, they used a handy long
to byte[]
conversion function in a widely used utility library. That function, in addition to allocating a byte[]
and stuffing a long
into it, also reverses the endianness of the long (the function appears to have been intended to convert long
values to network byte order).
Unfortunately, switching to a more appropriate hash function would've been a major change, so my fix for this was to change the hash function interface to take a pair of longs instead of a pair of byte arrays and have the hash function do the endianness reversal instead of doing it as a separate step (since the hash function was already shuffling bytes around, this didn't create additional work). Removing these unnecessary allocations made my employer more money annually than I've made in my life.
Finding a constant factor speedup isn't technically an algorithms question, but it's also something you see in algorithms interviews. As a follow-up to an algorithms question, I commonly get asked "can you make this faster?" The answer is to these often involves doing a simple optimization that will result in a constant factor improvement.
A concrete example that I've been asked twice in interviews is: you're storing IDs as ints, but you already have some context in the question that lets you know that the IDs are densely packed, so you can store them as a bitfield instead. The difference between the bitfield interview question and the real-world superfluous array is that the real-world existing solution is so far afield from the expected answer that you probably wouldn’t be asked to find a constant factor speedup. More likely, you would've failed the interview at that point.
To pick an example from another company, the configuration for BitFunnel, a search index used in Bing, is another example of an interview-level algorithms question3.
The full context necessary to describe the solution is a bit much for this blog post, but basically, there's a set of bloom filters that needs to be configured. One way to do this (which I'm told was being done) is to write a black-box optimization function that uses gradient descent to try to find an optimal solution. I'm told this always resulted in some strange properties and the output configuration always resulted in non-idealities which were worked around by making the backing bloom filters less dense, i.e. throwing more resources (and therefore money) at the problem.
To create a more optimized solution, you can observe that the fundamental operation in BitFunnel is equivalent to multiplying probabilities together, so, for any particular configuration, you can just multiply some probabilities together to determine how a configuration will perform. Since the configuration space isn't all that large, you can then put this inside a few for loops and iterate over the space of possible configurations and then pick out the best set of configurations. This isn't quite right because multiplying probabilities assumes a kind of independence that doesn't hold in reality, but that seems to work ok for the same reason that naive Bayesian spam filtering worked pretty well when it was introduced even though it incorrectly assumes the probability of any two words appearing in an email are independent. And if you want the full solution, you can work out the non-independent details, although that's probably beyond the scope of an interview.
Those are just three examples that came to mind, I run into this kind of thing all the time and could come up with tens of examples off the top of my head, perhaps more than a hundred if I sat down and tried to list every example I've worked on, certainly more than a hundred if I list examples I know of that someone else (or no one) has worked on. Both the examples in this post as well as the ones I haven’t included have these properties:
- The example could be phrased as an interview question
- If phrased as an interview question, you'd expect most (and probably) all people on the relevant team to get the right answer in the timeframe of an interview
- The cost savings from fixing the example is worth more annually than my lifetime earnings to date
- The example persisted for long enough that it's reasonable to assume that it wouldn't have been discovered otherwise
At the start of this post, we noted that people at big tech companies commonly claim that they have to do algorithms interviews since it's so costly to have inefficiencies at scale. My experience is that these examples are legion at every company I've worked for that does algorithms interviews. Trying to get people to solve algorithms problems on the job by asking algorithms questions in interviews doesn't work.
One reason is that even though big companies try to make sure that the people they hire can solve algorithms puzzles they also incentivize many or most developers to avoid deploying that kind of reasoning to make money.
Of the three solutions for the examples above, two are in production and one isn't. That's about my normal hit rate if I go to a random team with a diff and don't persistently follow up (as opposed to a team that I have reason to believe will be receptive, or a team that's asked for help, or if I keep pestering a team until the fix gets taken).
If you're very cynical, you could argue that it's surprising the success rate is that high. If I go to a random team, it's overwhelmingly likely that efficiency is in neither the team's objectives or their org's objectives. The company is likely to have spent a decent amount of effort incentivizing teams to hit their objectives -- what's the point of having objectives otherwise? Accepting my diff will require them to test, integrate, deploy the change and will create risk (because all deployments have non-zero risk). Basically, I'm asking teams to do some work and take on some risk to do something that's worthless to them. Despite incentives, people will usually take the diff, but they're not very likely to spend a lot of their own spare time trying to find efficiency improvements(and their normal work time will be spent on things that are aligned with the team's objectives)4.
Hypothetically, let's say a company didn't try to ensure that its developers could pass algorithms quizzes but did incentivize developers to use relatively efficient algorithms. I don't think any of the three examples above could have survived, undiscovered, for years nor could they have remained unfixed. Some hypothetical developer working at a company where people profile their code would likely have looked at the hottest items in the profile for the most computationally intensive library at the company. The "trick" for both isn't any kind of algorithms wizardry, it's just looking at all, which is something incentives can fix. The third example is less inevitable since there isn't a standard tool that will tell you to look at the problem. It would also be easy to try to spin the result as some kind of wizardry -- that example formed the core part of a paper that won "best paper award" at the top conference in its field (IR), but the reality is that the "trick" was applying high school math, which means the real trick was having enough time to look at places where high school math might be applicable to find one.
I actually worked at a company that used the strategy of "don't ask algorithms questions in interviews, but do incentivize things that are globally good for the company". During my time there, I only found one single fix that nearly meets the criteria for the examples above (if the company had more scale, it would've met all of the criteria, but due to the company's size, increases in efficiency were worth much less than at big companies -- much more than I was making at the time, but the annual return was still less than my total lifetime earnings to date).
I think the main reason that I only found one near-example is that enough people viewed making the company better as their job, so straightforward high-value fixes tended not exist because systems were usually designed such that they didn't really have easy to spot improvements in the first place. In the rare instances where that wasn't the case, there were enough people who were trying to do the right thing for the company (instead of being forced into obeying local incentives that are quite different from what's globally beneficial to the company) that someone else was probably going to fix the issue before I ever ran into it.
The algorithms/coding part of that company's interview (initial screen plus onsite combined) was easier than the phone screen at major tech companies and we basically didn't do a system design interview.
For a while, we tried an algorithmic onsite interview question that was on the hard side but in the normal range of what you might see in a BigCo phone screen (but still easier than you'd expect to see at an onsite interview). We stopped asking the question because every new grad we interviewed failed the question (we didn't give experienced candidates that kind of question). We simply weren't prestigious enough to get candidates who can easily answer those questions, so it was impossible to hire using the same trendy hiring filters that everybody else had. In contemporary discussions on interviews, what we did is often called "lowering the bar", but it's unclear to me why we should care how high of a bar someone can jump over when little (and in some cases none) of the job they're being hired to do involves jumping over bars. And, in the cases where you do want them to jump over bars, they're maybe 2" high and can easily be walked over.
When measured on actual productivity, that was the most productive company I've worked for. I believe the reasons for that are cultural and too complex to fully explore in this post, but I think it helped that we didn't filter out perfectly good candidates with algorithms quizzes and assumed people could pick that stuff up on the job if we had a culture of people generally doing the right thing instead of focusing on local objectives.
If other companies want people to solve interview-level algorithms problems on the job perhaps they could try incentivizing people to solve algorithms problems (when relevant). That could be done in addition to or even instead of filtering for people who can whiteboard algorithms problems.
Appendix: how did we get here?
Way back in the day, interviews often involved "trivia" questions. Modern versions of these might look like the following:
- What's MSI? MESI? MOESI? MESIF? What's the advantage of MESIF over MOESI?
- What happens when you throw in a destructor? What if it's C++11? What if a sub-object's destructor that's being called by a top-level destructor throws, which other sub-object destructors will execute? What if you throw during stack unwinding? Under what circumstances would that not cause
std::terminate
to get called?
I heard about this practice back when I was in school and even saw it with some "old school" companies. This was back when Microsoft was the biggest game in town and people who wanted to copy a successful company were likely to copy Microsoft. The most widely read programming blogger at the time (Joel Spolsky) was telling people they need to adopt software practice X because Microsoft was doing it and they couldn't compete without adopting the same practices. For example, in one of the most influential programming blog posts of the era, Joel Spolsky advocates for what he called the Joel test in part by saying that you have to do these things to keep up with companies like Microsoft:
A score of 12 is perfect, 11 is tolerable, but 10 or lower and you’ve got serious problems. The truth is that most software organizations are running with a score of 2 or 3, and they need serious help, because companies like Microsoft run at 12 full-time.
At the time, popular lore was that Microsoft asked people questions like the following (and I was actually asked one of these brainteasers during my on interview with Microsoft around 2001, along with precisely zero algorithms or coding questions):
- how would you escape from a blender if you were half an inch tall?
- why are manhole covers round?
- a windowless room has 3 lights, each of which is controlled by a switch outside of the room. You are outside the room. You can only enter the room once. How can you determine which switch controls which lightbulb?
Since I was interviewing during the era when this change was happening, I got asked plenty of trivia questions as well plenty of brainteasers (including all of the above brainteasers). Some other questions that aren't technically brainteasers that were popular at the time were Fermi problems. Another trend at the time was for behavioral interviews and a number of companies I interviewed with had 100% behavioral interviews with zero technical interviews.
Anyway, back then, people needed a rationalization for copying Microsoft-style interviews. When I asked people why they thought brainteasers or Fermi questions were good, the convenient rationalization people told me was usually that they tell you if a candidate can really think, unlike those silly trivia questions, which only tell if you people have memorized some trivia. What we really need to hire are candidates who can really think!
Looking back, people now realize that this wasn't effective and cargo culting Microsoft's every decision won't make you as successful as Microsoft because Microsoft's success came down to a few key things plus network effects, so copying how they interview can't possibly turn you into Microsoft. Instead, it's going to turn you into a company that interviews like Microsoft but isn't in a position to take advantage of the network effects that Microsoft was able to take advantage of.
For interviewees, the process with brainteasers was basically as it is now with algorithms questions, except that you'd review How Would You Move Mount Fuji before interviews instead of Cracking the Coding Interview to pick up a bunch of brainteaser knowledge that you'll never use on the job instead of algorithms knowledge you'll never use on the job.
Back then, interviewers would learn about questions specifically from interview prep books like "How Would You Move Mount Fuji?" and then ask them to candidates who learned the answers from books like "How Would You Move Mount Fuji?". When I talk to people who are ten years younger than me, they think this is ridiculous -- those questions obviously have nothing to do the job and being able to answer them well is much more strongly correlated with having done some interview prep than being competent at the job. Hillel Wayne has discussed how people come up with interview questions today (and I've also seen it firsthand at a few different companies) and, outside of groups that are testing for knowledge that's considered specialized, it doesn't seem all that different today.
At this point, we've gone through a few decades of programming interview fads, each one of which looks ridiculous in retrospect. Either we've finally found the real secret to interviewing effectively and have reasoned our way past whatever roadblocks were causing everybody in the past to use obviously bogus fad interview techniques, or we're in the middle of another fad, one which will seem equally ridiculous to people looking back a decade or two from now.
Without knowing anything about the effectiveness of interviews, at a meta level, since the way people get interview techniques is the same (crib the high-level technique from the most prestigious company around), I think it would be pretty surprising if this wasn't a fad. I would be less surprised to discover that current techniques were not a fad if people were doing or referring to empirical research or had independently discovered what works.
Inspired by a comment by Wesley Aptekar-Cassels, the last time I was looking for work, I asked some people how they checked the effectiveness of their interview process and how they tried to reduce bias in their process. The answers I got (grouped together when similar, in decreasing order of frequency were):
- Huh? We don't do that and/or why would we do that?
- We don't really know if our process is effective
- I/we just know that it works
- I/we aren't biased
- I/we would notice bias if it existed, which it doesn't
- Someone looked into it and/or did a study, but no one who tells me this can ever tell me anything concrete about how it was looked into or what the study's methodology was
Appendix: training
As with most real world problems, when trying to figure out why seven, eight, or even nine figure per year interview-level algorithms bugs are lying around waiting to be fixed, there isn't a single "root cause" you can point to. Instead, there's a kind of hedgehog defense of misaligned incentives. Another part of this is that training is woefully underappreciated.
We've discussed that, at all but one company I've worked for, there are incentive systems in place that cause developers to feel like they shouldn't spend time looking at efficiency gains even when a simple calculation shows that there are tens or hundreds of millions of dollars in waste that could easily be fixed. And then because this isn't incentivized, developers tend to not have experience doing this kind of thing, making it unfamiliar, which makes it feel harder than it is. So even when a day of work could return $1m/yr in savings or profit (quite common at large companies, in my experience), people don't realize that it's only a day of work and could be done with only a small compromise to velocity. One way to solve this latter problem is with training, but that's even harder to get credit for than efficiency gains that aren't in your objectives!
Just for example, I once wrote a moderate length tutorial (4500 words, shorter than this post by word count, though probably longer if you add images) on how to find various inefficiencies (how to use an allocation or CPU time profiler, how to do service-specific GC tuning for the GCs we use, how to use some tooling I built that will automatically find inefficiencies in your JVM or container configs, etc., basically things that are simple and often high impact that it's easy to write a runbook for; if you're at Twitter, you can read this at http://go/easy-perf). I've had a couple people who would've previously come to me for help with an issue tell me that they were able to debug and fix an issue on their own and, secondhand, I heard that a couple other people who I don't know were able to go off and increase the efficiency of their service. I'd be surprised if I’ve heard about even 10% of cases where this tutorial helped someone, so I'd guess that this has helped tens of engineers, and possibly quite a few more.
If I'd spent a week doing "real" work instead of writing a tutorial, I'd have something concrete, with quantifiable value, that I could easily put into a promo packet or performance review. Instead, I have this nebulous thing that, at best, counts as a bit of "extra credit". I'm not complaining about this in particular -- this is exactly the outcome I expected. But, on average, companies get what they incentivize. If they expect training to come from developers (as opposed to hiring people to produce training materials, which tends to be very poorly funded compared to engineering) but don't value it as much as they value dev work, then there's going to be a shortage of training.
I believe you can also see training under-incentivized in public educational materials due to the relative difficulty of monetizing education and training. If you want to monetize explaining things, there are a few techniques that seem to work very well. If it's something that's directly obviously valuable, selling a video course that's priced "very high" (hundreds or thousands of dollars for a short course) seems to work. Doing corporate training, where companies fly you in to talk to a room of 30 people and you charge $3k per head also works pretty well.
If you want to reach (and potentially help) a lot of people, putting text on the internet and giving it away works pretty well, but monetization for that works poorly. For technical topics, I'm not sure the non-ad-blocking audience is really large enough to monetize via ads (as opposed to a pay wall).
Just for example, Julia Evans can support herself from her zine income, which she's said has brought in roughly $100k/yr for the past two years. Someone who does very well in corporate training can pull that in with a one or two day training course and, from what I've heard of corporate speaking rates, some highly paid tech speakers can pull that in with two engagements. Those are significantly above average rates, especially for speaking engagements, but since we're comparing to Julia Evans, I don't think it's unfair to use an above average rate.
Appendix: misaligned incentive hedgehog defense, part 3
Of the three examples above, I found one on a team where it was clearly worth zero to me to do anything that was actually valuable to the company and the other two on a team where it valuable to me to do things that were good for the company, regardless of what they were. In my experience, that's very unusual for a team at a big company, but even on that team, incentive alignment was still quite poor. At one point, after getting a promotion and a raise, I computed the ratio of the amount of money my changes made the company vs. my raise and found that my raise was 0.03% of the money that I made the company, only counting easily quantifiable and totally indisputable impact to the bottom line. The vast majority of my work was related to tooling that had a difficult to quantify value that I suspect was actually larger than the value of the quantifiable impact, so I probably received well under 0.01% of the marginal value I was producing. And that's really an overestimate of how much I was incentivized I was to do the work -- at the margin, I strongly suspect that anything I did was worth zero to me. After the first $10m/yr or maybe $20m/yr, there's basically no difference in terms of performance reviews, promotions, raises, etc. Because there was no upside to doing work and there's some downside (could get into a political fight, could bring the site down, etc.), the marginal return to me of doing more than "enough" work was probably negative.
Some companies will give very large out-of-band bonuses to people regularly, but that work wasn't for a company that does a lot of that, so there's nothing the company could do to indicate that it valued additional work once someone did "enough" work to get the best possible rating on a performance review. From a mechanism design point of view, the company was basically asking employees to stop working once they did "enough" work for the year.
So even on this team, which was relatively well aligned with the company's success compared to most teams, the company's compensation system imposed a low ceiling on how well the team could be aligned.
This also happened in another way. As is common at a lot of companies, managers were given a team-wide budget for raises that was mainly a function of headcount, that was then doled out to team members in a zero-sum way. Unfortunately for each team member (at least in terms of compensation), the team pretty much only had productive engineers, meaning that no one was going to do particularly well in the zero-sum raise game. The team had very low turnover because people like working with good co-workers, but the company was applying one the biggest levers it has, compensation, to try to get people to leave the team and join less effective teams.
Because this is such a common setup, I've heard of managers at multiple companies who try to retain people who are harmless but ineffective to try to work around this problem. If you were to ask someone, abstractly, if the company wants to hire and retain people who are ineffective, I suspect they'd tell you no. But insofar as a company can be said to want anything, it wants what it incentivizes.
Related
- Downsides of cargo-culting trendy hiring practices
- Normalization of deviance
- Zvi Mowshowitz's on Moral Mazes, a book about how corporations have systemic issues that cause misaligned incentives at every level
- "randomsong" on on how it's possible to teach almost anybody to program. thematically related, the idea being that programming isn't as hard as a lot of programmers would like to believe
- Tanya Reilly on how "glue work" is poorly incentivized, training being poorly incentivized is arguably a special case of this
- Thomas Ptacek on using hiring filters that are decently correlated with job performance
- Michael Lynch on his personal experience of big company incentives
- An anonymous HN commenter on doing almost no work at Google, they say about 10% capacity, for six years and getting promoted
Thanks to Leah Hanson, Heath Borders, Lifan Zeng, Justin Findlay, Kevin Burke, @chordowl, Peter Alexander, Niels Olson, Kris Shamloo, Chip Thien, Yuri Vishnevsky, and Solomon Boulos for comments/corrections/discussion
- For one thing, most companies that copy the Google interview don't have that much scale. But even for companies that do, most people don't have jobs where they're designing high-scale algorithms (maybe they did at Google circa 2003, but from what I've seen at three different big tech companies, most people's jobs are pretty light on algorithms work). [return]
-
Real is in quotes because I've passed a number of interviews for reasons outside of the interview process. Maybe I had a very strong internal recommendation that could override my interview performance, maybe someone read my blog and assumed that I can do reasonable work based on my writing, maybe someone got a backchannel reference from a former co-worker of mine, or maybe someone read some of my open source code and judged me on that instead of a whiteboard coding question (and as far as I know, that last one has only happened once or twice). I'll usually ask why I got a job offer in cases where I pretty clearly failed the technical interview, so I have a collection of these reasons from folks.
The reason it's arguably zero is that the only software interview where I inarguably got a "real" interview and was coming in cold was at Google, but that only happened because the interviewers that were assigned interviewed me for the wrong ladder -- I was interviewing for a hardware position, but I was being interviewed by software folks, so I got what was basically a standard software interview except that one interviewer asked me some questions about state machine and cache coherence (or something like that). After they realized that they'd interviewed me for the wrong ladder, I had a follow-up phone interview from a hardware engineer to make sure I wasn't totally faking having worked at a hardware startup from 2005 to 2013. It's possible that I failed the software part of the interview and was basically hired on the strength of the follow-up phone screen.
Note that this refers only to software -- I'm actually pretty good at hardware interviews. At this point, I'm pretty out of practice at hardware and would probably need a fair amount of time to ramp up on an actual hardware job, but the interviews are a piece of cake for me. One person who knows me pretty well thinks this is because I "talk like a hardware engineer" and both say things that make hardware folks think I'm legit as well as say things that sound incredibly stupid to most programmers in a way that's more about shibboleths than actual knowledge or skills.
[return] -
This one is a bit harder than you'd expect to get in a phone screen, but it wouldn't be out of line in an onsite interview (although a friend of mine once got a Google Code Jam World Finals question in a phone interview with Google, so you might get something this hard or harder, depending on who you draw as an interviewer).
BTW, if you're wondering what my friend did when they got that question, it turns out they actually knew the answer because they'd seen and attempted the problem during Google Code Jam. They didn't get the right answer at the time, but they figured it out later just for fun. However, my friend didn't think it was reasonable to give that as a phone screen questions and asked the interviewer for another question. The interviewer refused, so my friend failed the phone screen. At the time, I doubt there were more than a few hundred people in the world who would've gotten the right answer to the question in a phone screen and almost all of them probably would've realized that it was an absurd phone screen question. After failing the interview, my friend ended up looking for work for almost six months before passing an interview for a startup where he ended up building a number of core systems (in terms of both business impact and engineering difficulty). My friend is still there after the mid 10-figure IPO -- the company understands how hard it would be to replace this person and treats them very well. None of the other companies that interviewed this person even wanted to hire them at all and they actually had a hard time getting a job.
[return] - Outside of egregious architectural issues that will simply cause a service to fall over, the most common way I see teams fix efficiency issues is to ask for more capacity. Some companies try to counterbalance this in some way (e.g., I've heard that at FB, a lot of the teams that work on efficiency improvements report into the capacity org, which gives them the ability to block capacity requests if they observe that a team has extreme inefficiencies that they refuse to fix), but I haven't personally worked in an environment where there's an effective system fix to this. Google had a system that was intended to address this problem that, among other things, involved making headcount fungible with compute resources, but I've heard that was rolled back in favor of a more traditional system for reasons. [return]
Fleen Book Corner: Good, Good Boys
The Adventure Zone: Here Be Gerblins is maybe the book that is least for me that I’ve ever looked forward to. I believe that I’ve mentioned that I don’t listen to any of the McElroy-related media empire — not because I’m not interested, but because I know that I would get sucked into listening to every. single. damn. one. they do, or are associated with. Every month or so I can go by YouTube and see which bits are attracting all the new animatics, and I quite enjoyed their guest turn on Bubble, but that’s as far as I can go. I have work, people.
But TAZ:HBG has brought me right up to the precipice. If I fall into a McElhole, it’s because of this book.
Which is weird, because it shouldn’t have worked. A story made up on the fly (and remade) by four different people (brothers Justin, Travis, Griffin, and dad Clint), and adapted to story form by two (Clint and artist Carey Pietsch) should be a mess. Griffin surely knows the pain of every dungeon master who’s ever lived as the players derail everything you’ve planned and go off in a million directions … and when those players are known for digressive goofery and several thousand tangents per second? There’s no way to get a single, coherent narrative from that starting point.
Except they do. Credit to Griffin for clearly having an idea of where he wanted the story to go and accounting for all the fuckery his family could throw at it. Further credit to Clint and Pietsch for finding a way to pare down to that story, while still coming up with means to include the best fourth-wall breaks, character introductions, scene shifts, and the flavor of the gaming sessions¹. It’s straddled the line between playing a game and what the lives of the collectively-created characters are like in the game rather nicely.
But that’s not why you should read the book. You should read the book for one exchange, during the last of the increasingly-difficult boss battles, when who the McElroys are comes through. They’ve spent 200 pages playing characters who are willing to tolerate each other, but who range between self-regard, self-delusion, self-interest, and self-aggrandizement.
Magnus (human, fighter, played by Travis) is cocksure, rushes in without thinking, and generally makes things worse. Taako (elf, wizard, played by Justin) full of himself, not above a bit of thievery, and generally makes things worse. Merle (dwarf, cleric, played by Clint) is grouchy, doesn’t like his family or the mission, and generally makes things worse.
Then the Big Bad threatens a town that they don’t care about at all. Taako’s fled to a place of marginal safety and for once, Magnus hesitates.
Magnus: I’m not leaving with all these people here!!
Merle: Magnus … you can’t save everybody.
Magnus: Maybe not — but that doesn’t mean you can’t try.
And there it is. Despite playing a blundering jerk for hours and hours, Travis can’t help but find a place to inject the fundamental decency for which the McElroy boys are known. It’s going to cost Magnus his life, it’s going to derail the game (and the podcast series)², and Travis’s dad reacts the only way he can.
Merle: Well … shit.
And then they’re off, transformed from adventurers to heroes. Even Taako finds a way to to care — despite insisting that he doesn’t care — and act to help Magnus and Merle. They’ll still be jerks, they’ll still try to scam their way through life, but they’ve turned a corner without really intending to. Griffin may have set the conditions that made it possible, but when Magnus, Merle, and Taako could have cut and run, Travis, Clint, and Justin decided that they wouldn’t.
It would be a hard thing for one author to pull off — heck, it’s taken masters of character growth like Randy Milholland and Meredith Gran hundreds of strips over years to accomplish such redemptive arcs — and four people working occasionally in parallel (but just as often at cross) purposes pulled it off in the space of a minute. Pietsch conveyed the entire thing in three pages, and the centerpiece, that emotional turn from Magnus and Merle in three panels.
And that’s why this book that isn’t for me, one that I looked forward to from a remove, was ultimately worth it. Because in and around all the goofs and sniping and shit-talking and messing with the DM and each other, little grace notes pervade. You can be a bit of a dick, and still want to save the helpless. It’s a hell of a message.
Oh, and the whole thing with the sshhkxxx? That’s one great story hook you came up with, Griffin. Nice one.
Spam of the day:
100’S celebs and artists like Ms. Hudson are eating this fruit every day. With this amazing fruit your body will enter Ketosis without changing diets or gym you can lose 4lbs a day
Okay, EMT hat on here — ketosis is your body’s metabolic mechanism to desperately attempt to keep your brain alive when you’re starving it of glucose. It is not a means to — as the subject line of this spam promised — get abs.
_______________
¹ My favorites, in no particular order:
- DM Griffin appears in inset panels when he interacts with the story; on his first appearance, the players panic and he has to calm them.
- Scrolls appear to introduce new characters and their defining abilities.
- What must have been wildly looping, heavily descriptive role-playing (Well, I say ______. Okay then, I say _____ in response, and suck it!) is constructed into naturalish dialogue.
- Running gags about game mechanics appear, as do repeated hints by Clint about popular songs; at first, the boys mock him out of character, but by the end, they’re referencing Oklahoma and The Girl From Ipanema in-character and it works.
² Unless Griffin can make with the DM magic, fudging rules and consequences to keep the story going that is.
Australian cricket ball tampering: officials arrive for talks in South Africa – as it happened
- Updates as Australia wakes up to reports the coach will quit
- On a cricket field the captain is responsible | Matthew Engel
- ‘Say it ain’t so, Steve’ – at least cricket still matters
As Sutherland heads into crunch meetings, that’s going to be it from us for now, but stay with the Guardian for updates on any developments. Thanks for following along! Passing on to the UK, where a new live blog is under way.
Related: Cricket Australia set to wield axe over ball-tampering scandal – live!
We have just received confirmation from a Cricket Australia spokesman – CEO James Sutherland just landed in Johannesburg, at around 7am local time.
When asked about Lehmann’s future, the spokesman said CA won’t comment on rumour and speculation.
Steve Smith looks set to lose a significant chunk of money as a result of this scandal, and this piece from Fox Sports steps us through the sums of what could be a $5m loss.
Smith is the best paid player in the national team, on a salary of $2m a year ($14,000 per test match). He’s also the highest paid Australian in the Indian Premier League (along with David Warner), earning $2.4m a year from the Rajasthan Royals.
In the absence of any real news, here’s some fake news.
The Australian advertising industry website AdNews have been fooled by a fake post from satirical website the Betoota Advocate, which claimed Cricket Australia was suing them over a podcast.
While Steve Smith awaits the arrival of James Sutherland, back home the embattled captain has just been mentioned in a Melbourne court hearing for former AFL player Jake King.
According to the Australian, the presiding magistrate mentioned Smith when discussing King’s character witnesses.
Caffy Sutcliffe has emailed in to echo a sentiment, voiced by Paul Connolly about Australian fans’ slow drift away from the national team. “It was during [Michael] Clarke’s captaincy that I started to go off the game,” she writes. “I couldn’t bring myself to watch one minute of any test, so ashamed as I was of the Australian team. I was justified in my dislike, of Warner in particular, and Smith as captain, and wanted nothing to do with international men’s cricket at all.
“This is a new low point. It’s stumps for me, and a very, very long tea break. One from which I may never return.”
More emails! Bob Elliott has written in to point out the links between cheating and a profit-driven sporting culture. “National cricket is a business and not a sport,” he says. “It is about profits and bottom line. This requires employees (otherwise known as players) to win (often at any cost).”
Richard Woods is calling for former player Michael Klinger to be picked as captain. “Like Langer, he would be completely untainted by current events,” he writes. “As a player, no one could doubt his worth in any form of the game. He would command respect for everyone while those responsible clean out the Augean stables.”
The last time Sutherland fronted the press, it got a bit heated. Here’s footage of two journalists in Melbourne on Sunday in a shoving match after a press conference.
For far better video content, watch our explainer on the history of ball tampering.
If earlier reports are true, James Sutherland will have just landed in Johannesburg. It’s 5.17 in the morning there, with AAP reporting earlier that his flight was scheduled to land at 5am.
The fallout from the incident has also created a rift in the team, according to Sydney’s Daily Telegraph.
The paper have reported that bowlers Mitchell Starc and Josh Hazlewood are angry at Smith for suggesting they knew of the plan as part of the much discussed “leadership group”. One of the pair reportedly contacted the player’s union, the Australian Cricketers Association, to ask for help in clearing their names.
Who is in line to replace Lehmann, Smith and Warner if, as expected, all three resign or are suspended?
Justin Langer, the current head coach of Western Australia, will be “fast-tracked” into the job, according to The Australian. Langer had been tipped to take over from Lehmann in 2019.
Just over six months ago, Tim Paine was preparing to start his new life in management at Kookaburra. Today, he's going to captain the Australian Test team. Extraordinary. #AUSvSA
Breaking. Smith and Warner stand down as Captain and Vice Captain.
Tim Paine new captain.
I know. Who?
But we return now, unfortunately to the division and disgrace.
The Australian prime minister, Malcolm Turnbull, has again blasted the cheating that took place in South Africa.
Turnbull also says sledging "should have no place on a cricket pitch". "Some of the sledging and some of the shocking conduct that we've seen is part of the process of review that's going to be undertaken." #auspol
Here’s the first reader email of the day!
Susan O’Sullivan writes:
Let’s shift the focus to the best of Australian cricket – the Australian women’s cricket team. Sanitarium should sponsor them and Qantas should shift a large part of sponsorship there
HAT TRICK! pic.twitter.com/391UnmDmAK
Fox Sports reporter Tom Morris has told the channel that Lehmann’s resignation is coming, and will be a disappointing end to his reign after he oversaw this year’s Ashes win.
“He’s not a stupid person, Darren Lehmann, he can read the tea leaves,” Morris said. “It looks as if he won’t be the coach going forward.”
Readers may remember that back in January, Smith faced accusations of ball tampering during the ODI against England, which he denied.
Critics suggested he had wiped lip balm or a similar substance on the ball, but Smith insisted it was just spit.
Related: Steve Smith denies ball-tampering after Australia captain is seen wiping lip
Here’s the schedule for today, for those confused as to what will happen to Darren Lehmann and when:
This could be the final straw, writes Paul Connolly, hot off the press with a fantastic meditation on this week’s scandal and how it reflects a broader issue in Australian sporting culture.
Like many others I’ve spoken to, I simply became turned off by their ugly, envelope-pushing aggression which hasn’t abated ... I’ve played a lot of sport in my life and despite never competing at a particularly high level I became well acquainted with the hyper-aggressive Australian male, the one for whom competitiveness and arseholery seem to be mutually inclusive.
Related: Ball tampering scandal could be Australian fans' tipping point | Paul Connolly
How are the Australian papers covering the scandal as it rolls on to day three?
Most NewsCorp papers are running an arresting front page of former all-rounder Trevor Chappell, grim-faced and in a thin grey henley, declaring that he is “no longer the most hated man in cricket”.
lol Trevor Chappell looking like the ghost of cricket past pic.twitter.com/OwVvuPyXG6
Good morning. Here's today's front page of the Herald https://t.co/eEH8m1plEu pic.twitter.com/jsNwb7YtwT
Related: 'Clown Under': papers roast Steve Smith and Australian team after cricket scandal
South African broadcaster SuperSport have denied a widely-shared report that they were tipped off about Australia’s ball tampering ahead of time.
On Saturday, SuperSport captured the crucial footage of Cameron Bancroft’s ill-fated attempt to hide his sticky tape down his pants, prompting Smith’s admission of cheating.
If you’d like to read what may be the definitive takedown from a former player, go no further than Jason Gillespie’s opinion piece from yesterday.
Australian cricket will survive this and provided there is some honesty with the camp, it will hopefully emerge in better shape. New leaders will rise up and take the side forward. But it will not be Smith and it will not be Warner.
Related: Steve Smith must go after scandal that has torched Australia’s reputation | Jason Gillespie
On Fox Sports Australia, we’re hearing that as of last night, Lehmann was determined to stay on.
Commentators are in the dark, but according to NewsCorp journalist Richard Earle “something major” must have changed the head coach’s mind.
We’re still waiting on more information on Lehmann’s purported resignation, but it’s undeniable that pressure has been mounting on the head coach for days.
A range of current and former players, from Australia and abroad, have called his position untenable.
"They've got no option but to sack Smith, Warner and Lehmann."
Simon Katich has his say on the ball tampering saga with @GerardWhateley. #SAvAUS #SENTestCricket pic.twitter.com/H5l4VgW74P
Slept on it...Lehmann, Saker & the leaderships groups jobs are untenable!
They’ve disgraced a great cricketing nation & Test cricket!
Bud you know nothing in professional sport is done without the consent of your captain and coach... Tough times ahead.
In case you missed it, have a read of Barney Ronay’s excellent take on the saga from this morning.
Darren Lehmann ... has overseen an infantilised team culture where such a plot was so easily conceived and where fear of being discovered by good old Boof was clearly not much of an issue. Nothing less than the sack will do for Lehmann. He should be profoundly embarrassed already, although past conduct suggests this may not be the case.
Related: ‘Say it ain’t so Steve’ – at least this scandal shows cricket still matters | Barney Ronay
In one of the lone voices of dissent, Australian cricketer Moises Henriques has defended Steve Smith.
Henriques took to Twitter to share his alternate theory – that there was no “leadership group” meeting and no clear plan. He speculated that Smith was covering for batsman Cameron Bancroft in “10 minutes of panic”.
In my uneducated opinion, I dare say there was never a senior players meeting to discuss cheating - Smith made that up to take the heat of a young Cameron Bancroft not realising the outrage that would follow.
Ps. Not saying no one was aware of Cameron doing it, just highly doubt there was a ‘senior players meeting’ to decide to cheat. I think it was the captain attempting to protect a young player. They had 10 mins of panic between end of play & press conference.
Cricket Australia’s major sponsor, the airline Qantas, has called on the body to “do the right thing” at an event at the Australian High Commission in London.
According to the Australian newspaper, Qantas CEO Alan Joyce made a strong statement at a function where all anybody could talk about was Smith and the ball tampering scandal.
Erstwhile vice-captain David Warner may have played a bigger role in the ball tampering plan than previously realised, Australia’s Sydney Morning Herald are reporting.
Warner, who like Smith was stood down for the rest of the series, has been labelled “the central character” by the SMH’s Chris Barrett.
We’re trying to get hold of Cricket Australia to confirm the overnight report from the UK’s Daily Telegraph.
However their phones, understandably, are running quite hot.
Hello there. Australia is waking up to reports that Darren Lehmann, the coach, will resign from his position in the next 24 hours, as the ball tampering scandal that has rocked the sport appears set to claim its first victim.
The coach’s position has come under intense scrutiny since captain Steve Smith’s admission that his team conspired to tamper with the ball during the third Test in Cape Town.
Programming book recommendations and anti-recommendations
There are a lot of “12 CS books every programmer must read” lists floating around out there. That's nonsense. The field is too broad for almost any topic to be required reading for all programmers, and even if a topic is that important, people's learning preferences differ too much for any book on that topic to be the best book on the topic for all people.
This is a list of topics and books where I've read the book, am familiar enough with the topic to say what you might get out of learning more about the topic, and have read other books and can say why you'd want to read one book over another.
Algorithms / Data Structures / Complexity
Why should you care? Well, there's the pragmatic argument: even if you never use this stuff in your job, most of the best paying companies will quiz you on this stuff in interviews. On the non-bullshit side of things, I find algorithms to be useful in the same way I find math to be useful. The probability of any particular algorithm being useful for any particular problem is low, but having a general picture of what kinds of problems are solved problems, what kinds of problems are intractable, and when approximations will be effective, is often useful.
McDowell; Cracking the Coding Interview
Some problems and solutions, with explanations, matching the level of questions you see in entry-level interviews at Google, Facebook, Microsoft, etc. I usually recommend this book to people who want to pass interviews but not really learn about algorithms. It has just enough to get by, but doesn't really teach you the why behind anything. If you want to actually learn about algorithms and data structures, see below.
Dasgupta, Papadimitriou, and Vazirani; Algorithms
Everything about this book seems perfect to me. It breaks up algorithms into classes (e.g., divide and conquer or greedy), and teaches you how to recognize what kind of algorithm should be used to solve a particular problem. It has a good selection of topics for an intro book, it's the right length to read over a few weekends, and it has exercises that are appropriate for an intro book. Additionally, it has sub-questions in the middle of chapters to make you reflect on non-obvious ideas to make sure you don't miss anything.
I know some folks don't like it because it's relatively math-y/proof focused. If that's you, you'll probably prefer Skiena.
Skiena; The Algorithm Design Manual
The longer, more comprehensive, more practical, less math-y version of Dasgupta. It's similar in that it attempts to teach you how to identify problems, use the correct algorithm, and give a clear explanation of the algorithm. Book is well motivated with “war stories” that show the impact of algorithms in real world programming.
CLRS; Introduction to Algorithms
This book somehow manages to make it into half of these “N books all programmers must read” lists despite being so comprehensive and rigorous that almost no practitioners actually read the entire thing. It's great as a textbook for an algorithms class, where you get a selection of topics. As a class textbook, it's nice bonus that it has exercises that are hard enough that they can be used for graduate level classes (about half the exercises from my grad level algorithms class were pulled from CLRS, and the other half were from Kleinberg & Tardos), but this is wildly impractical as a standalone introduction for most people.
Just for example, there's an entire chapter on Van Emde Boas trees. They're really neat -- it's a little surprising that a balanced-tree-like structure with O(lg lg n)
insert, delete, as well as find, successor, and predecessor is possible, but a first introduction to algorithms shouldn't include Van Emde Boas trees.
Kleinberg & Tardos; Algorithm Design
Same comments as for CLRS -- it's widely recommended as an introductory book even though it doesn't make sense as an introductory book. Personally, I found the exposition in Kleinberg to be much easier to follow than in CLRS, but plenty of people find the opposite.
Demaine; Advanced Data Structures
This is a set of lectures and notes and not a book, but if you want a coherent (but not intractably comprehensive) set of material on data structures that you're unlikely to see in most undergraduate courses, this is great. The notes aren't designed to be standalone, so you'll want to watch the videos if you haven't already seen this material.
Okasaki; Purely Functional Data Structures
Fun to work through, but, unlike the other algorithms and data structures books, I've yet to be able to apply anything from this book to a problem domain where performance really matters.
For a couple years after I read this, when someone would tell me that it's not that hard to reason about the performance of purely functional lazy data structures, I'd ask them about part of a proof that stumped me in this book. I'm not talking about some obscure super hard exercise, either. I'm talking about something that's in the main body of the text that was considered too obvious to the author to explain. No one could explain it. Reasoning about this kind of thing is harder than people often claim.
Dominus; Higher Order Perl
A gentle introduction to functional programming that happens to use Perl. You could probably work through this book just as easily in Python or Ruby.
If you keep up with what's trendy, this book might seem a bit dated today, but only because so many of the ideas have become mainstream. If you're wondering why you should care about this "functional programming" thing people keep talking about, and some of the slogans you hear don't speak to you or are even off-putting (types are propositions, it's great because it's math, etc.), give this book a chance.
Levitin; Algorithms
I ordered this off amazon after seeing these two blurbs: “Other learning-enhancement features include chapter summaries, hints to the exercises, and a detailed solution manual.” and “Student learning is further supported by exercise hints and chapter summaries.” One of these blurbs is even printed on the book itself, but after getting the book, the only self-study resources I could find were some yahoo answers posts asking where you could find hints or solutions.
I ended up picking up Dasgupta instead, which was available off an author's website for free.
Mitzenmacher & Upfal; Probability and Computing: Randomized Algorithms and Probabilistic Analysis
I've probably gotten more mileage out of this than out of any other algorithms book. A lot of randomized algorithms are trivial to port to other applications and can simplify things a lot.
The text has enough of an intro to probability that you don't need to have any probability background. Also, the material on tails bounds (e.g., Chernoff bounds) is useful for a lot of CS theory proofs and isn't covered in the intro probability texts I've seen.
Sipser; Introduction to the Theory of Computation
Classic intro to theory of computation. Turing machines, etc. Proofs are often given at an intuitive, “proof sketch”, level of detail. A lot of important results (e.g, Rice's Theorem) are pushed into the exercises, so you really have to do the key exercises. Unfortunately, most of the key exercises don't have solutions, so you can't check your work.
For something with a more modern topic selection, maybe see Aurora & Barak.
Bernhardt; Computation
Covers a few theory of computation highlights. The explanations are delightful and I've watched some of the videos more than once just to watch Bernhardt explain things. Targeted at a general programmer audience with no background in CS.
Kearns & Vazirani; An Introduction to Computational Learning Theory
Classic, but dated and riddled with errors, with no errata available. When I wanted to learn this material, I ended up cobbling together notes from a couple of courses, one by Klivans and one by Blum.
Operating Systems
Why should you care? Having a bit of knowledge about operating systems can save days or week of debugging time. This is a regular theme on Julia Evans's blog, and I've found the same thing to be true of my experience. I'm hard pressed to think of anyone who builds practical systems and knows a bit about operating systems who hasn't found their operating systems knowledge to be a time saver. However, there's a bias in who reads operating systems books -- it tends to be people who do related work! It's possible you won't get the same thing out of reading these if you do really high-level stuff.
Silberchatz, Galvin, and Gagne; Operating System Concepts
This was what we used at Wisconsin before the comet book became standard. I guess it's ok. It covers concepts at a high level and hits the major points, but it's lacking in technical depth, details on how things work, advanced topics, and clear exposition.
Cox, Kasshoek, and Morris; xv6
This book is great! It explains how you can actually implement things in a real system, and it comes with its own implementation of an OS that you can play with. By design, the authors favor simple implementations over optimized ones, so the algorithms and data structures used are often quite different than what you see in production systems.
This book goes well when paired with a book that talks about how more modern operating systems work, like Love's Linux Kernel Development or Russinovich's Windows Internals.
Arpaci-Dusseau and Arpaci-Dusseau; Operating Systems: Three Easy Pieces
Nice explanation of a variety of OS topics. Goes into much more detail than any other intro OS book I know of. For example, the chapters on file systems describe the details of multiple, real, filessytems, and discusses the major implementation features of ext4
. If I have one criticism about the book, it's that it's very *nix focused. Many things that are described are simply how things are done in *nix and not inherent, but the text mostly doesn't say when something is inherent vs. when it's a *nix implementation detail.
Love; Linux Kernel Development
The title can be a bit misleading -- this is basically a book about how the Linux kernel works: how things fit together, what algorithms and data structures are used, etc. I read the 2nd edition, which is now quite dated. The 3rd edition has some updates, but introduced some errors and inconsistencies, and is still dated (it was published in 2010, and covers 2.6.34). Even so, it's a nice introduction into how a relatively modern operating system works.
The other downside of this book is that the author loses all objectivity any time Linux and Windows are compared. Basically every time they're compared, the author says that Linux has clearly and incontrovertibly made the right choice and that Windows is doing something stupid. On balance, I prefer Linux to Windows, but there are a number of areas where Windows is superior, as well as areas where there's parity but Windows was ahead for years. You'll never find out what they are from this book, though.
Russinovich, Solomon, and Ionescu; Windows Internals
The most comprehensive book about how a modern operating system works. It just happens to be about Windows. Coming from a *nix background, I found this interesting to read just to see the differences.
This is definitely not an intro book, and you should have some knowledge of operating systems before reading this. If you're going to buy a physical copy of this book, you might want to wait until the 7th edition is released (early in 2017).
Downey; The Little Book of Semaphores
Takes a topic that's normally one or two sections in an operating systems textbook and turns it into its own 300 page book. The book is a series of exercises, a bit like The Little Schemer, but with more exposition. It starts by explaining what semaphore is, and then has a series of exercises that builds up higher level concurrency primitives.
This book was very helpful when I first started to write threading/concurrency code. I subscribe to the Butler Lampson school of concurrency, which is to say that I prefer to have all the concurrency-related code stuffed into a black box that someone else writes. But sometimes you're stuck writing the black box, and if so, this book has a nice introduction to the style of thinking required to write maybe possibly not totally wrong concurrent code.
I wish someone would write a book in this style, but both lower level and higher level. I'd love to see exercises like this, but starting with instruction-level primitives for a couple different architectures with different memory models (say, x86 and Alpha) instead of semaphores. If I'm writing grungy low-level threading code today, I'm overwhelmingly likely to be using c++11
threading primitives, so I'd like something that uses those instead of semaphores, which I might have used if I was writing threading code against the Win32
API. But since that book doesn't exist, this seems like the next best thing.
I've heard that Doug Lea's Concurrent Programming in Java is also quite good, but I've only taken a quick look at it.
Computer architecture
Why should you care? The specific facts and trivia you'll learn will be useful when you're doing low-level performance optimizations, but the real value is learning how to reason about tradeoffs between performance and other factors, whether that's power, cost, size, weight, or something else.
In theory, that kind of reasoning should be taught regardless of specialization, but my experience is that comp arch folks are much more likely to “get” that kind of reasoning and do back of the envelope calculations that will save them from throwing away a 2x or 10x (or 100x) factor in performance for no reason. This sounds obvious, but I can think of multiple production systems at large companies that are giving up 10x to 100x in performance which are operating at a scale where even a 2x difference in performance could pay a VP's salary -- all because people didn't think through the performance implications of their design.
Hennessy & Patterson; Computer Architecture: A Quantitative Approach
This book teaches you how to do systems design with multiple constraints (e.g., performance, TCO, and power) and how to reason about tradeoffs. It happens to mostly do so using microprocessors and supercomputers as examples.
New editions of this book have substantive additions and you really want the latest version. For example, the latest version added, among other things, a chapter on data center design, and it answers questions like, how much opex/capex is spent on power, power distribution, and cooling, and how much is spent on support staff and machines, what's the effect of using lower power machines on tail latency and result quality (bing search results are used as an example), and what other factors should you consider when designing a data center.
Assumes some background, but that background is presented in the appendices (which are available online for free).
Shen & Lipasti: Modern Processor Design
Presents most of what you need to know to architect a high performance Pentium Pro (1995) era microprocessor. That's no mean feat, considering the complexity involved in such a processor. Additionally, presents some more advanced ideas and bounds on how much parallelism can be extracted from various workloads (and how you might go about doing such a calculation). Has an unusually large section on value prediction, because the authors invented the concept and it was still hot when the first edition was published.
For pure CPU architecture, this is probably the best book available.
Hill, Jouppi, and Sohi, Readings in Computer Architecture
Read for historical reasons and to see how much better we've gotten at explaining things. For example, compare Amdahl's paper on Amdahl's law (two pages, with a single non-obvious graph presented, and no formulas), vs. the presentation in a modern textbook (one paragraph, one formula, and maybe one graph to clarify, although it's usually clear enough that no extra graph is needed).
This seems to be worse the further back you go; since comp arch is a relatively young field, nothing here is really hard to understand. If you want to see a dramatic example of how we've gotten better at explaining things, compare Maxwell's original paper on Maxwell's equations to a modern treatment of the same material. Fun if you like history, but a bit of slog if you're just trying to learn something.
Algorithmic game theory / auction theory / mechanism design
Why should you care? Some of the world's biggest tech companies run on ad revenue, and those ads are sold through auctions. This field explains how and why they work. Additionally, this material is useful any time you're trying to figure out how to design systems that allocate resources effectively.1
In particular, incentive compatible mechanism design (roughly, how to create systems that provide globally optimal outcomes when people behave in their own selfish best interest) should be required reading for anyone who designs internal incentive systems at companies. If you've ever worked at a large company that "gets" this and one that doesn't, you'll see that the company that doesn't get it has giant piles of money that are basically being lit on fire because the people who set up incentives created systems that are hugely wasteful. This field gives you the background to understand what sorts of mechanisms give you what sorts of outcomes; reading case studies gives you a very long (and entertaining) list of mistakes that can cost millions or even billions of dollars.
Krishna; Auction Theory
The last time I looked, this was the only game in town for a comprehensive, modern, introduction to auction theory. Covers the classic second price auction result in the first chapter, and then moves on to cover risk aversion, bidding rings, interdependent values, multiple auctions, asymmetrical information, and other real-world issues.
Relatively dry. Unlikely to be motivating unless you're already interested in the topic. Requires an understanding of basic probability and calculus.
Steighlitz; Snipers, Shills, and Sharks: eBay and Human Behavior
Seems designed as an entertaining introduction to auction theory for the layperson. Requires no mathematical background and relegates math to the small print. Covers maybe, 1/10th of the material of Krishna, if that. Fun read.
Crampton, Shoham, and Steinberg; Combinatorial Auctions
Discusses things like how FCC spectrum auctions got to be the way they are and how “bugs” in mechanism design can leave hundreds of millions or billions of dollars on the table. This is one of those books where each chapter is by a different author. Despite that, it still manages to be coherent and I didn't mind reading it straight through. It's self-contained enough that you could probably read this without reading Krishna first, but I wouldn't recommend it.
Shoham and Leyton-Brown; Multiagent Systems: Algorithmic, Game-Theoretic, and Logical Foundations
The title is the worst thing about this book. Otherwise, it's a nice introduction to algorithmic game theory. The book covers basic game theory, auction theory, and other classic topics that CS folks might not already know, and then covers the intersection of CS with these topics. Assumes no particular background in the topic.
Nisan, Roughgarden, Tardos, and Vazirani; Algorithmic Game Theory
A survey of various results in algorithmic game theory. Requires a fair amount of background (consider reading Shoham and Leyton-Brown first). For example, chapter five is basically Devanur, Papadimitriou, Saberi, and Vazirani's JACM paper, Market Equilibrium via a Primal-Dual Algorithm for a Convex Program, with a bit more motivation and some related problems thrown in. The exposition is good and the result is interesting (if you're into that kind of thing), but it's not necessarily what you want if you want to read a book straight through and get an introduction to the field.
Misc
Beyer, Jones, Petoff, and Murphy; Site Reliability Engineering
A description of how Google handles operations. Has the typical Google tone, which is off-putting to a lot of folks with a “traditional” ops background, and assumes that many things can only be done with the SRE model when they can, in fact, be done without going full SRE.
For a much longer description, see this 22 page set of notes on Google's SRE book.
Fowler, Beck, Brant, Opdyke, and Roberts; Refactoring
At the time I read it, it was worth the price of admission for the section on code smells alone. But this book has been so successful that the ideas of refactoring and code smells have become mainstream.
Steve Yegge has a great pitch for this book:
When I read this book for the first time, in October 2003, I felt this horrid cold feeling, the way you might feel if you just realized you've been coming to work for 5 years with your pants down around your ankles. I asked around casually the next day: "Yeah, uh, you've read that, um, Refactoring book, of course, right? Ha, ha, I only ask because I read it a very long time ago, not just now, of course." Only 1 person of 20 I surveyed had read it. Thank goodness all of us had our pants down, not just me.
...
If you're a relatively experienced engineer, you'll recognize 80% or more of the techniques in the book as things you've already figured out and started doing out of habit. But it gives them all names and discusses their pros and cons objectively, which I found very useful. And it debunked two or three practices that I had cherished since my earliest days as a programmer. Don't comment your code? Local variables are the root of all evil? Is this guy a madman? Read it and decide for yourself!
Demarco & Lister, Peopleware
This book seemed convincing when I read it in college. It even had all sorts of studies backing up what they said. No deadlines is better than having deadlines. Offices are better than cubicles. Basically all devs I talk to agree with this stuff.
But virtually every successful company is run the opposite way. Even Microsoft is remodeling buildings from individual offices to open plan layouts. Could it be that all of this stuff just doesn't matter that much? If it really is that important, how come companies that are true believers, like Fog Creek, aren't running roughshod over their competitors?
This book agrees with my biases and I'd love for this book to be right, but the meta evidence makes me want to re-read this with a critical eye and look up primary sources.
Drummond; Renegades of the Empire
This book explains how Microsoft's aggressive culture got to be the way it is today. The intro reads:
Microsoft didn't necessarily hire clones of Gates (although there were plenty on the corporate campus) so much as recruiter those who shared some of Gates's more notable traits -- arrogance, aggressiveness, and high intelligence.
…
Gates is infamous for ridiculing someone's idea as “stupid”, or worse, “random”, just to see how he or she defends a position. This hostile managerial technique invariably spread through the chain of command and created a culture of conflict.
…
Microsoft nurtures a Darwinian order where resources are often plundered and hoarded for power, wealth, and prestige. A manager who leaves on vacation might return to find his turf raided by a rival and his project put under a different command or canceled altogether
On interviewing at Microsoft:
“What do you like about Microsoft?” “Bill kicks ass”, St. John said. “I like kicking ass. I enjoy the feeling of killing competitors and dominating markets”.
…
He was unsure how he was doing and thought he stumbled then asked if he was a "people person". "No, I think most people are idiots", St. John replied.
These answers were exactly what Microsoft was looking for. They resulted in a strong offer and an aggresive courtship.
On developer evangalism at Microsoft:
At one time, Microsoft evangelists were also usually chartered with disrupting competitors by showing up at their conferences, securing positions on and then tangling standards commitees, and trying to influence the media.
…
"We're the group at Microsoft whose job is to fuck Microsoft's competitors"
Read this book if you're considering a job at Microsoft. Although it's been a long time since the events described in this book, you can still see strains of this culture in Microsoft today.
Bilton; Hatching Twitter
An entertaining book about the backstabbing, mismangement, and random firings that happened in Twitter's early days. When I say random, I mean that there were instances where critical engineers were allegedly fired so that the "decider" could show other important people that current management was still in charge.
I don't know folks who were at Twitter back then, but I know plenty of folks who were at the next generation of startups in their early days and there are a couple of companies where people had eerily similar experiences. Read this book if you're considering a job at a trendy startup.
Galenson; Old Masters and Young Geniuses
This book is about art and how productivity changes with age, but if its thesis is valid, it probably also applies to programming. Galenson applies statistics to determine the "greatness" of art and then uses that to draw conclusions about how the productivty of artists change as they age. I don't have time to go over the data in detail, so I'll have to remain skeptical of this until I have more free time, but I think it's interesting reading even for a skeptic.
Math
Why should you care? From a pure ROI perspective, I doubt learning math is “worth it” for 99% of jobs out there. AFAICT, I use math more often than most programmers, and I don't use it all that often. But having the right math background sometimes comes in handy and I really enjoy learning math. YMMV.
Bertsekas; Introduction to Probability
Introductory undergrad text that tends towards intuitive explanations over epsilon-delta rigor. For anyone who cares to do more rigorous derivations, there are some exercises at the back of the book that go into more detail.
Has many exercises with available solutions, making this a good text for self-study.
Ross; A First Course in Probability
This is one of those books where they regularly crank out new editions to make students pay for new copies of the book (this is presently priced at a whopping $174 on Amazon)2. This was the standard text when I took probability at Wisconsin, and I literally cannot think of a single person who found it helpful. Avoid.
Brualdi; Introductory Combinatorics
Brualdi is a great lecturer, one of the best I had in undergrad, but this book was full of errors and not particularly clear. There have been two new editions since I used this book, but according to the Amazon reviews the book still has a lot of errors.
For an alternate introductory text, I've heard good things about Camina & Lewis's book, but I haven't read it myself. Also, Lovasz is a great book on combinatorics, but it's not exactly introductory.
Apostol; Calculus
Volume 1 covers what you'd expect in a calculus I + calculus II book. Volume 2 covers linear algebra and multivariable calculus. It covers linear algebra before multivariable calculus, which makes multi-variable calculus a lot easier to understand.
It also makes a lot of sense from a programming standpoint, since a lot of the value I get out of calculus is its applications to approximations, etc., and that's a lot clearer when taught in this sequence.
This book is probably a rough intro if you don't have a professor or TA to help you along. The Spring SUMS series tends to be pretty good for self-study introductions to various areas, but I haven't actually read their intro calculus book so I can't actually recommend it.
Stewart; Calculus
Another one of those books where they crank out new editions with trivial changes to make money. This was the standard text for non-honors calculus at Wisconsin, and the result of that was I taught a lot of people to do complex integrals with the methods covered in Apostol, which are much more intuitive to many folks.
This book takes the approach that, for a type of problem, you should pattern match to one of many possible formulas and then apply the formula. Apostol is more about teaching you a few tricks and some intuition that you can apply to a wide variety of problems. I'm not sure why you'd buy this unless you were required to for some class.
Hardware basics
Why should you care? People often claim that, to be a good programmer, you have to understand every abstraction you use. That's nonsense. Modern computing is too complicated for any human to have a real full-stack understanding of what's going on. In fact, one reason modern computing can accomplish what it does is that it's possible to be productive without having a deep understanding of much of the stack that sits below the level you're operating at.
That being said, if you're curious about what sits below software, here are a few books that will get you started.
Nisan & Shocken; nand2tetris
If you only want to read one single thing, this should probably be it. It's a “101” level intro that goes down to gates and Boolean logic. As implied by the name, it takes you from NAND gates to a working tetris program.
Roth; Fundamentals of Logic Design
Much more detail on gates and logic design than you'll see in nand2tetris. The book is full of exercises and appears to be designed to work for self-study. Note that the link above is to the 5th edition. There are newer, more expensive, editions, but they don't seem to be much improved, have a lot of errors in the new material, and are much more expensive.
Weste; Harris, and Bannerjee; CMOS VLSI Design
One level below Boolean gates, you get to VLSI, a historical acronym (very large scale integration) that doesn't really have any meaning today.
Broader and deeper than the alternatives, with clear exposition. Explores the design space (e.g., the section on adders doesn't just mention a few different types in an ad hoc way, it explores all the tradeoffs you can make. Also, has both problems and solutions, which makes it great for self study.
Kang & Leblebici; CMOS Digital Integrated Circuits
This was the standard text at Wisconsin way back in the day. It was hard enough to follow that the TA basically re-explained pretty much everything necessary for the projects and the exams. I find that it's ok as a reference, but it wasn't a great book to learn from.
Compared to West et al., Weste spends a lot more effort talking about tradeoffs in design (e.g., when creating a parallel prefix tree adder, what does it really mean to be at some particular point in the design space?).
Pierret; Semiconductor Device Fundamentals
One level below VLSI, you have how transistors actually work.
Really beautiful explanation of solid state devices. The text nails the fundamentals of what you need to know to really understand this stuff (e.g., band diagrams), and then uses those fundamentals along with clear explanations to give you a good mental model of how different types of junctions and devices work.
Streetman & Bannerjee; Solid State Electronic Devices
Covers the same material as Pierret, but seems to substitute mathematical formulas for the intuitive understanding that Pierret goes for.
Ida; Engineering Electromagnetics
One level below transistors, you have electromagnetics.
Two to three times thicker than other intro texts because it has more worked examples and diagrams. Breaks things down into types of problems and subproblems, making things easy to follow. For self-study, A much gentler introduction than Griffiths or Purcell.
Shanley; Pentium Pro and Pentium II System Architecture
Unlike the other books in this section, this book is about practice instead of theory. It's a bit like Windows Internals, in that it goes into the details of a real, working, system. Topics include hardware bus protocols, how I/O actually works (e.g., APIC), etc.
The problem with a practical introduction is that there's been an exponential increase in complexity ever since the 8080. The further back you go, the easier it is to understand the most important moving parts in the system, and the more irrelevant the knowledge. This book seems like an ok compromise in that the bus and I/O protocols had to handle multiprocessors, and many of the elements that are in modern systems were in these systems, just in a simpler form.
Not covered
Of the books that I've liked, I'd say this captures at most 25% of the software books and 5% of the hardware books. On average, the books that have been left off the list are more specialized. This list is also missing many entire topic areas, like PL, practical books on how to learn languages, networking, etc.
The reasons for leaving off topic areas vary; I don't have any PL books listed because I don't read PL books. I don't have any networking books because, although I've read a couple, I don't know enough about the area to really say how useful the books are. The vast majority of hardware books aren't included because they cover material that you wouldn't care about unless you were a specialist (e.g., Skew-Tolerant Circuit Design or Ultrafast Optics). The same goes for areas like math and CS theory, where I left off a number of books that I think are great but have basically zero probability of being useful in my day-to-day programming life, e.g., Extremal Combinatorics. I also didn't include books I didn't read all or most of, unless I stopped because the book was atrocious. This means that I don't list classics I haven't finished like SICP and The Little Schemer, since those book seem fine and I just didn't finish them for one reason or another.
This list also doesn't include many books on history and culture, like Inside Intel or Masters of Doom. I'll probably add more at some point, but I've been trying an experiment where I try to write more like Julia Evans (stream of consciousness, fewer or no drafts). I'd have to go back and re-read the books I read 10+ years ago to write meaningful comments, which doesn't exactly fit with the experiment. On that note, since this list is from memory and I got rid of almost all of my books a couple years ago, I'm probably forgetting a lot of books that I meant to add.
_If you liked this, you might also like Thomas Ptacek's Application Security Reading List or this list of programming blogs, which is written in a similar style_
_Thanks to @tytrdev for comments/corrections/discussion.
- Also, if you play board games, auction theory explains why fixing game imbalance via an auction mechanism is non-trivial and often makes the game worse. [return]
- I talked to the author of one of these books. He griped that the used book market destroys revenue from textbooks after a couple years, and that authors don't get much in royalties, so you have to charge a lot of money and keep producing new editions every couple of years to make money. That griping goes double in cases where a new author picks up a book classic book that someone else originally wrote, since the original author often has a much larger share of the royalties than the new author, despite doing no work no the later editions. [return]
Some programming blogs to consider reading
This is one of those “N technical things every programmer must read” lists, except that “programmer” is way too broad a term and the styles of writing people find helpful for them are too different for any such list to contain a non-zero number of items (if you want the entire list to be helpful to everyone). So here's a list of some things you might want to read, and why you might (or might not) want to read them.
Aleksey Shipilev
If you want to understand how the JVM really works, this is one of the best resources on the internet.
Bruce Dawson
Performance explorations of a Windows programmer. Often implicitly has nice demonstrations of tooling that has no publicly available peer on Linux.
Chip Huyen
A mix of summaries of ML conferences, data analyses (e.g., on interview data posted to glassdoor or compensation data posted to levels.fyi), and generaly commentary on the industry.
One of the rare blogs that has data-driven position pieces about the industry.
Chris Fenton
Computer related projects, by which I mean things like reconstructing the Cray-1A and building mechanical computers. Rarely updated, presumably due to the amount of work that goes into the creations, but almost always interesting.
The blog posts tend to be high-level, more like pitch decks than design docs, but there's often source code available if you want more detail.
Cindy Sridharan
More active on Twitter than on her blog, but has posts that review papers as well as some on "big" topics, like distributed tracing and testing in production.
Dan McKinley
A lot of great material on how engineering companies should be run. He has a lot of ideas that sound like common sense, e.g., choose boring technology, until you realize that it's actually uncommon to find opinions that are so sensible.
Mostly distilled wisdom (as opposed to, say, detailed explanations of code).
Eli Bendersky
I think of this as “the C++ blog”, but it's much wider ranging that that. It's too wide ranging for me to sum up, but if I had to commit to a description I might say that it's a collection of deep dives into various topics, often (but not always) relatively low-level, along with short blurbs about books, often (but not always) technical.
The book reviews tend to be easy reading, but the programming blog posts are often a mix of code and exposition that really demands your attention; usually not a light read.
Erik Sink
I think Erik has been the most consistently insightful writer about tech culture over the past 20 years. If you look at people who were blogging back when he started blogging, much of Steve Yegge's writing holds up as well as Erik's, but Steve hasn't continued writing consistently.
If you look at popular writers from that era, I think they generally tend to not really hold up very well.
Fabian Giesen
Covers a wide variety of technical topics. Emphasis on computer architecture, compression, graphics, and signal processing, but you'll find many other topics as well.
Posts tend towards being technically intense and not light reading and they usually explain concepts or ideas (as opposed to taking sides and writing opinion pieces).
Fabien Sanglard
In depth techincal dives on game related topics, such as this readthrough of the Doom source code, this history of Nvidia GPU architecture, or this read of a business card raytracer.
Fabrice Bellard
Not exactly a blog, but every time a new project appears on the front page, it's amazing. Some examples are QEMU, FFMPEG, a 4G LTE base station that runs on a PC, a JavaScript PC emulator that can boot Linux, etc.
Fred Akalin
Explanations of CS-related math topics (with a few that aren't directly CS related).
Gary Bernhardt
Another “not exactly a blog”, but it's more informative than most blogs, not to mention more entertaining. This is the best “blog” on the pervasive brokenness of modern software that I know of.
Jaana Dogan
rakyll.org has posts on Go, some of which are quite in depth, e.g., this set of notes on the Go generics proposal and Jaana's medium blog has some posts on Go as well as posts on various topics in distributed systems.
Also, Jaana's Twitter has what I think of as "intellectually honest critiques of the industry", which I think is unusual for critiques of the industry on Twitter. It's more typical to see people scoring points at the expense of nuance or even being vaugely in the vicinity of correctness, which is why I think it's worth calling out these honest critiques.
Jamie Brandon
I'm so happy that I managed to convince Jamie that, given his preferences, it would make sense to take a crack at blogging full-time to support himself. Since Jamie started taking donations until today, this blog has been an absolute power house with posts like this series on problems with SQL, this series on streaming systems, great work on technical projects like dida and imp, etc.
It remains to be seen whether or not Jamie will be able to convince me to try blogging as a full-time job.
Janet Davis
This is the story of how a professor moved from Grinnel to Whitman and started a CS program from scratch. The archives are great reading if you're interested in how organizations form or CS education.
Jeff Preshing
Mostly technical content relating to C++ and Python, but also includes topics that are generally useful for programmers, such as read-modify-write operations, fixed-point math, and memory models.
Jessica Kerr
Jessica is probably better known for her talks than her blog? Her talks are great! My favorite is probably this talk with explains different concurrency models in an easy to understand way, but the blog also has a lot of material I like.
As is the case with her talks, the diagrams often take a concept and clarify it, making something that wasn't obvious seem very obvious in retrospect.
John Regehr
I think of this as the “C is harder than you think, even if you think C is really hard” blog, although the blog actually covers a lot more than that. Some commonly covered topics are fuzzing, compiler optimization, and testing in general.
Posts tend to be conceptual. When there are code examples, they're often pretty easy to read, but there are also examples of bizzaro behavior that won't be easy to skim unless you're someone who knows the C standard by heart.
Juho Snellman
A lot of posts about networking, generally written so that they make sense even with minimal networking background. I wish more people with this kind of knowledge (in depth knowledge of systems, not just networking knowledge in particular) would write up explanations for a general audience. Also has interesting non-networking content, like this post on Finnish elections.
Julia Evans
AFAICT, the theme is “things Julia has learned recently”, which can be anything from Huffman coding to how to be happy when working in a remote job. When the posts are on a topic I don't already know, I learn something new. When they're on a topic I know, they remind me that the topic is exciting and contains a lot of wonder and mystery.
Many posts have more questions than answers, and are more of a live-blogged exploration of a topic than an explanation of the topic.
Karla Burnett
A mix of security-related topics and explanations of practical programming knowledge. This article on phishing, which includes a set of fun case studies on how effective phising can be, even after people take anti-phishing training, is an example of a security post. This post on printing out text via tracert. This post on writing an SSH client and this post on some coreutils puzzles are examples of practical programming explanations.
Although the blog is security oriented, posts are written for a general audience and don't assume specific expertise in security.
Kate Murphy
Mostly small, self-contained explorations like, what's up with this Python integer behavior, how do you make a git blow up with a simple repo, or how do you generate hash collisions in Lua?
Kavya Joshi
I generally prefer technical explanations in text over video, but her exposition is so clear that I'm putting these talks in this list of blogs. Some examples include an explanation of the go race detector, simple math that's handy for performance modeling, and time.
Kyle Kingsbury
90% of Kyle's posts are explanations of distributed systems testing, which expose bugs in real systems that most of us rely on. The other 10% are musings on programming that are as rigorous as Kyle's posts on distributed systems. Possibly the most educational programming blog of all time.
For those of us without a distributed systems background, understanding posts often requires a bit of Googling, despite the extensive explanations in the posts. Most new posts are now at jepsen.io
Laura Lindzey
Very infrequently updated (on the order of once a year) with explanations of things Laura has been working on, from Oragami PCB to Ice-Penetrating Radar.
Laurie Tratt
This blog has been going since 2004 and its changed over the years. Recently, it's had some of the best posts on benchmarking around:
-
VM performance, part 1
- Thoroughly refutes the idea that you can run a language VM for some warmup period and then take some numbers when they become stable
- VM performance, part 2
-
Why not use minimum times when benchmarking
- "Everyone" who's serious about performance knows this and it's generally considered too obvious to write up, but this is still a widely used technique in benchmarking even though it's only appropriate in limited circumstances
The blog isn't purely technical, this blog post on advice is also stellar. If those posts don't sound interesting to you, it's worth checking out the archives to see if some of the topics Lawrence used to write about more frequently are to your taste.
Marc Brooker
A mix of theory and wisdom from a distributed systems engineer on EBS at Amazon. The theory posts tend to be relatively short and easy to swallow; not at all intimidating, as theory sometimes is.
Marek Majkowski
This used to be a blog about random experiments Marek was doing, like this post on bitsliced SipHash. Since Marek joined Cloudflare, this has turned into a list of things Marek has learned while working in Cloudflare's networking stack, like this story about debugging slow downloads.
Posts tend to be relatively short, but with enough technical specifics that they're not light reads.
Nicole Express
Explorations on old systems, often gaming related. Some exmaples are this post on collision detection in Alf for the Sega Master System, this post on getting decent quality output from composite video, and this post on the Neo Geo CDZ.
Nikita Prokopov
Nikita has two blogs, both on related topics. The main blog has long-form articles, often how about modern software is terrible. THen there's grumpy.website, which gives examples of software being terrible.
Nitsan Wakart
More than you ever wanted to know about writing fast code for the JVM, from GV affects data structures to the subtleties of volatile reads.
Posts tend to involve lots of Java code, but the takeaways are often language agnostic.
Oona Raisanen
Adventures in signal processing. Everything from deblurring barcodes to figuring out what those signals from helicopters mean. If I'd known that signals and systems could be this interesting, I would have paid more attention in class.
Paul Khuong
Some content on Lisp, and some on low-level optimizations, with a trend towards low-level optimizations.
Posts are usually relatively long and self-contained explanations of technical ideas with very little fluff.
Rachel Kroll
Years of debugging stories from a long-time SRE, along with stories about big company nonsense. Many of the stories come from Lyft, Facebook, and Google. They're anonymized, but if you know about the companies, you can tell which ones are which.
The degree of anonymization often means that the stories won't really make sense unless you're familiar with the operation of systems similar to the ones in the stories.
Sophie Haskins
A blog about restoring old "pizza box" computers, with posts that generally describe the work that goes into getting these machines working again.
An example is the HP 712 ("low cost" PA-RISC workstations that went for roughly $5k to $15k in 1994 dollars, which ended up doomed due to the Intel workstation onslaught that started with the Pentium Pro in 1995), where the restoration process is described here in part 1 and then here in part 2.
Vyacheslav Egorov
In-depth explanations on how V8 works and how various constructs get optimized by a compiler dev on the V8 team. If I knew compilers were this interesting, I would have taken a compilers class back when I was in college.
Often takes topics that are considered hard and explains them in a way that makes them seem easy. Lots of diagrams, where appropriate, and detailed exposition on all the tricky bits.
whitequark
Her main site has to a variety of interesting tools she's made or worked on, many of which are FPGA or open hardware related, but some of which are completely different. Whitequark's lab notebook has a really wide variety of different results, from things like undocumented hardware quirks, to fairly serious home chemistry experiments, to various tidbits about programming and hardware development (usually low level, but not always).
She's also fairly active on twitter, with some commentary on hardware/firmware/low-level programming combined with a set of diverse topics that's too broad to easily summarize.
Yossi Kreinin
Mostly dormant since the author started doing art, but the archives have a lot of great content about hardware, low-level software, and general programming-related topics that aren't strictly programming.
90% of the time, when I get the desire to write a post about a common misconception software folks have about hardware, Yossi has already written the post and taken a lot of flak for it so I don't have to :-).
I also really like Yossi's career advice, like this response to Patrick McKenzie and this post on how managers get what they want and not what they ask for.
He's active on Twitter, where he posts extremely cynical and snarky takes on management and the industry.
This blog?
Common themes include:
- This thing that's often considered easy is harder than you might think
- This thing that's often considered hard is easier than you might think
- This obvious fact is not obvious at all
- Humans are human: humans make mistakes, and systems must be designed to account for that
- Computers will have faults, and systems must be designed to account for that
- Is it just me, or is stuff really broken?
- Hey! Look at this set of papers! Let's talk about their context and why they're interesting
The end
This list also doesn't include blogs that mostly aren't about programming, so it doesn't include, for example, Ben Kuhn's excellent blog.
Anyway, that's all for now, but this list is pretty much off the top of my head, so I'll add more as more blogs come to mind. I'll also keep this list updated with what I'm reading as I find new blogs. Please please please suggest other blogs I might like, and don't assume that I already know about a blog because it's popular. Just for example, I had no idea who either Jeff Atwood or Zed Shaw were until a few years ago, and they were probably two of the most well known programming bloggers in existence. Even with centralized link aggregators like HN and reddit, blog discovery has become haphazard and random with the decline of blogrolls and blogging as a dialogue, as opposed to the current practice of blogging as a monologue. Also, please don't assume that I don't want to read something just because it's different from the kind of blog I normally read. I'd love to read more from UX or front-end folks; I just don't know where to find that kind of thing!
Last update: 2021-07
Archive
Here are some blogs I've put into an archive section because they rarely or never update.
Alex Clemmer
This post on why making a competitor to Google search is a post in classic Alex Clemmer style. The post looks at a position that's commonly believed (web search isn't all that hard and someone should come up with a better Google) and explains why that's not an obviously correct position. That's also a common theme of his comments elsewhere, such as these comments on, stack ranking at MS, implementing POSIX on Windows, the size of the Windows codebase, Bond, and Bing.
He's sort of a modern mini-MSFT, in that it's incisive commentary on MS and MS related ventures.
Allison Kaptur
Explorations of various areas, often Python related, such as this this series on the Python interpreter and this series on the CPython peephole optimizer. Also, thoughts on broader topics like debugging and learning.
Often detailed, with inline code that's meant to be read and understood (with the help of exposition that's generally quite clear).
David Dalrymple
A mix of things from writing a 64-bit kernel from scratch shortly after learning assembly to a high-level overview of computer systems. Rarely updated, with few posts, but each post has a lot to think about.
EPITA Systems Lab
Low-level. A good example of a relatively high-level post from this blog is this post on the low fragmentation heap in Windows. Posts like how to hack a pinball machine and how to design a 386 compatible dev board are typical.
Posts are often quite detailed, with schematic/circuit diagrams. This is relatively heavy reading and I try to have pen and paper handy when I'm reading this blog.
Greg Wilson
Write-ups of papers that (should) have an impact on how people write software, like this paper on what causes failures in distributed systems or this paper on what makes people feel productive. Not updated much, but Greg still blogs on his personal site.
The posts tend to be extended abstracts that tease you into reading the paper, rather than detailed explanations of the methodology and results.
Gustavo Duarte
Explanations of how Linux works, as well as other low-level topics. This particular blog seems to be on hiatus, but "0xAX" seems to have picked up the slack with the linux-insides project.
If you've read Love's book on Linux, Duarte's explanations are similar, but tend to be more about the idea and less about the implementation. They're also heavier on providing diagrams and context. "0xAX" is a lot more focused on walking through the code than either Love or Duarte.
Huon Wilson
Explanations of various Rust-y things, from back when Huon was working on Rust. Not updated much anymore, but the content is still great for someone who's interested in technical tidbits related to Rust.
Kamal Marhubi
Technical explorations of various topics, with a systems-y bent. Kubernetes. Git push. Syscalls in Rust. Also, some musings on programming in general.
The technical explorations often get into enough nitty gritty detail that this is something you probably want to sit down to read, as opposed to skim on your phone.
Mary Rose Cook
Lengthy and very-detailed explanations of technical topics, mixed in with a wide variety of other posts.
The selection of topics is eclectic, and explained at a level of detail such that you'll come away with a solid understanding of the topic. The explanations are usually fine grained enough that it's hard to miss what's going on, even if you're a beginner programmer.
Rebecca Frankel
As far as I know, Rebecca doesn't have a programming blog, but if you look at her apparently off-the-cuff comments on other people's posts as a blog, it's one of the best written programming blogs out there. She used to be prolific on Piaw's Buzz (and probably elsewhere, although I don't know where), and you occasionally see comments elsewhere, like on this Steve Yegge blog post about brilliant engineers1. I wish I could write like that.
Russell Smith
Homemade electronics projects from vim on a mechanical typewriter to building an electrobalance to proof spirits.
Posts tend to have a fair bit of detail, down to diagrams explaining parts of circuits, but the posts aren't as detailed as specs. But there are usually links to resources that will teach you enough to reproduce the project, if you want.
RWT
I find the archives to be fun reading for insight into what people were thinking about microprocessors and computer architecture over the past two decades. It can be a bit depressing to see that the same benchmarking controversies we had 15 years ago are being repeated today, sometimes with the same players. If anything, I'd say that the average benchmark you see passed around today is worse than what you would have seen 15 years ago, even though the industry as a whole has learned a lot about benchmarking since then.
walpurgusriot
The author of walpurgisriot seems to have abanoned the github account and moved on to another user name (and a squatter appears to have picked up her old account name), but this used to be a semi-frequently updated blog with a combination of short explorations on programming and thoughts on the industry. On pure quality of prose, this is one of the best tech blogs I've ever read; the technical content and thoughts on the industry are great as well.
This post was inspired by the two posts Julia Evans has on blogs she reads and by the Chicago undergraduate mathematics bibliography, which I've found to be the most useful set of book reviews I've ever encountered.
Thanks to Bartłomiej Filipek and Sean Barrett, Michel Schniz, Neil Henning, and Lindsey Kuper for comments/discussion/corrections.
-
Quote follows below, since I can see from my analytics data that relatively few people click any individual link, and people seem especially unlikely to click a link to read a comment on a blog, even if the comment is great:
The key here is "principally," and that I am describing motivation, not self-evaluation. The question is, what's driving you? What gets you working? If its just trying to show that you're good, then you won't be. It has to be something else too, or it won't get you through the concentrated decade of training it takes to get to that level.
Look at the history of the person we're all presuming Steve Yegge is talking about. He graduated (with honors) in 1990 and started at Google in 1999. So he worked a long time before he got to the level of Google's star. When I was at Google I hung out on Sunday afternoons with a similar superstar. Nobody else was reliably there on Sunday; but he always was, so I could count on having someone to talk to. On some Sundays he came to work even when he had unquestionably legitimate reasons for not feeling well, but he still came to work. Why didn't he go home like any normal person would? It wasn't that he was trying to prove himself; he'd done that long ago. What was driving him?
The only way I can describe it is one word: fury. What was he doing every Sunday? He was reviewing various APIs that were being proposed as standards by more junior programmers, and he was always finding things wrong with them. What he would talk about, or rather, rage about, on these Sunday afternoons was always about some idiocy or another that someone was trying make standard, and what was wrong with it, how it had to be fixed up, etc, etc. He was always in a high dudgeon over it all.
What made him come to work when he was feeling sick and dizzy and nobody, not even Larry and Sergey with their legendary impatience, not even them, I mean nobody would have thought less of him if he had just gone home & gone to sleep? He seemed to be driven, not by ambition, but by fear that if he stopped paying attention, something idiotically wrong (in his eyes) might get past him, and become the standard, and that was just unbearable, the thought made him so incoherently angry at the sheer wrongness of it, that he had to stay awake and prevent it from happening no matter how legitimately bad he was feeling at the time.
It made me think of Paul Graham's comment: "What do I mean by good people? One of the best tricks I learned during our startup was a rule for deciding who to hire. Could you describe the person as an animal?... I mean someone who takes their work a little too seriously; someone who does what they do so well that they pass right through professional and cross over into obsessive.
What it means specifically depends on the job: a salesperson who just won't take no for an answer; a hacker who will stay up till 4:00 AM rather than go to bed leaving code with a bug in it; a PR person who will cold-call New York Times reporters on their cell phones; a graphic designer who feels physical pain when something is two millimeters out of place."
I think a corollary of this characterization is that if you really want to be "an animal," what you have cultivate in yourself is partly ambition, but it is partly also self-knowledge. As Paul Graham says, there are different kinds of animals. The obsessive graphic designer might be unconcerned about an API that is less than it could be, while the programming superstar might pass by, or create, a terrible graphic design without the slightest twinge of misgiving.
Therefore, key question is: are you working on the thing you care about most? If its wrong, is it unbearable to you? Nothing but deep seated fury will propel you to the level of a superstar. Getting there hurts too much; mere desire to be good is not enough. If its not in you, its not in you. You have to be propelled by elemental wrath. Nothing less will do.
Or it might be in you, but just not in this domain. You have to find what you care about, and not just what you care about, but what you care about violently: you can't fake it.
(Also, if you do have it in you, you still have to choose your boss carefully. No matter how good you are, it may not be trivial to find someone you can work for. There's more to say here; but I'll have to leave it for another comment.)
Another clarification of my assertion "if you're wondering if you're good, then you're not" should perhaps be said "if you need reassurance from someone else that you're good, then you're not." One characteristic of these "animals" is that they are such obsessive perfectionists that their own internal standards so far outstrip anything that anyone else could hold them to, that no ordinary person (i.e. ordinary boss) can evaluate them. As Steve Yegge said, they don't go for interviews. They do evaluate each other -- at Google the superstars all reviewed each other's code, reportedly brutally -- but I don't think they cared about the judgments of anyone who wasn't in their circle or at their level.
I agree with Steve Yegge's assertion that there are an enormously important (small) group of people who are just on another level, and ordinary smart hardworking people just aren't the same. Here's another way to explain why there should be a quantum jump -- perhaps I've been using this discussion to build up this idea: its the difference between people who are still trying to do well on a test administered by someone else, and the people who have found in themselves the ability to grade their own test, more carefully, with more obsessive perfectionism, than anyone else could possibly impose on them.
School, for all it teaches, may have one bad lasting effect on people: it gives them the idea that good people get A's on tests, and better ones get A+'s on tests, and the very best get A++'s. Then you get the idea that you go out into the real world, and your boss is kind of super-professor, who takes over the grading of the test. Joel Spolsky is accepting that role, being boss as super-professor, grading his employees tests for them, telling them whether they are good.
But the problem is that in the real world, the very most valuable, most effective people aren't the ones who are trying to get A+++'s on the test you give them. The very best people are the ones who can make up their own test with harder problems on it than you could ever think of, and you'd have to have studied for the same ten years they have to be able even to know how to grade their answers.
That's a problem, incidentally, with the idea of a meritocracy. School gives you an idea of a ladder of merit that reaches to the top. But it can't reach all the way to the top, because someone has to measure the rungs. At the top you're not just being judged on how high you are on the ladder. You're also being judged on your ability to "grade your own test"; that is to say, your trustworthiness. People start asking whether you will enforce your own standards even if no one is imposing them on you. They have to! because at the top people get given jobs with the kind of responsibility where no one can possibly correct you if you screw up. I'm giving you an image of someone who is working himself sick, literally, trying grade everyone else's work. In the end there is only so much he can do, and he does want to go home and go to bed sometimes. That means he wants people under him who are not merely good, but can be trusted not to need to be graded. Somebody has to watch the watchers, and in the end, the watchers have to watch themselves.
Preview: Copa America semifinals — Can Peru, Paraguay stop an Argentina-Chile final?

Must-See NHL TV: A Cheat Sheet on the Games to Watch for the Rest of the Season
It’s a busy night on the NHL schedule, one of just 17 left in the regular season. With tight playoff races in both conferences and first place in three of the four divisions still up for grabs, there’s going to be compelling action just about every night the rest of the way.
For some of you, that’s great — you can pretty much get comfortable on the couch now and settle in for the next few weeks. But if work, family, or other commitments mean you need to be a little more selective in your hockey viewing, now is the time to plan ahead. And because I’m here to help, I went through every night of the remaining schedule to figure out which games will be must-see TV and which ones you can go ahead and skip. Get out your calendars and a highlighter or two, because here we go.
Thursday, March 26 — 11 games
The can’t-miss matchup: Coyotes at Sabres — It says something about this tank-fest of a season that this is the night’s most intriguing matchup, and that something probably isn’t good, but here we are. The Sabres and Coyotes are going down to the wire for 30th place, and they play twice in the next five nights. Will either team try? Will they both start their backup goalies? Will any Sabre who scores a goal be immediately beaten over the head with a shovel by Tim Murray? I honestly can’t wait.
Other good options: Ducks at Bruins and Rangers at Senators (the two teams fighting for the East’s final wild-card spot both host Presidents’ Trophy candidates); Predators at Lightning (a game that would have passed for a Stanley Cup preview six weeks ago, and might still be); Sharks at Wings (what may be a showdown between the two best available coaches this offseason); Kings at Islanders (L.A.’s tough road trip continues); Canadiens at Jets (yet another Presidents’ Trophy candidate trying to squash a wild-card hopeful).
No thanks: Panthers at Maple Leafs — Is Rob Tallas suiting up again? No? Pass.
Entertainment quotient: 8/10 — Lots going on tonight. We’re off to a good start.
Friday, March 27 — Three games
The can’t-miss matchup: Flames at Wild — Two teams scrambling for the West’s final playoff spots meet, and fans of the teams chasing them know what that means: guaranteed three-point game night!
Other good options: Blue Jackets at Blackhawks (I know, I know, but at least one is a playoff team).
No thanks: Stars at Oilers — Two non-playoff teams makes this one meaningless, although the potential for a 9-8 final is vaguely intriguing.
Entertainment quotient: 2/10 — Welp. They can’t all be winners.
Saturday, March 28 — 13 games
The can’t-miss matchup: Kings at Wild — The defending champs are on shaky ground to get back into the playoffs, and they’ll face one of the wild-card teams they’re chasing during a brutal five-game road trip.
Other good options: Rangers at Bruins (an Original Six matchup between teams headed in opposite directions); Lightning at Red Wings (a possible first-round preview of a series we’d all agree to call the Steve Yzerman Cup); Ducks at Islanders (two very good teams that usually play entertaining games); Predators at Capitals (in which Washington fans spend the whole game wishing they could someday draft a rookie as good as Filip Forsberg).
No thanks: Devils at Hurricanes — Two teams that are bad, but not bad enough to be in contention for 30th. By the way, fair warning: Both teams are going to show up a lot in this section.
Entertainment quotient: 8/10 — Lots of games, some of them excellent, most of them decent. What else were you going to do on a Saturday night?
Sunday, March 29 — Eight games
The can’t-miss matchup: Panthers at Senators — I know, I’m as surprised as you are. But these teams are chasing the East’s final wild-card spot, and there’s only room for one. This is probably as close to a must-win as you can get in March, especially for the Panthers.
Other good options: Red Wings at Islanders (two solid Eastern playoff teams); Blackhawks at Jets (a sneaky important Central matchup); Capitals at Rangers (looking more and more like a first-round preview); Flames at Predators (facing off for the “teams I was most inexcusably wrong about” championship).
No thanks: Ducks at Devils — It’s the awesome team with shaky goaltending versus the shaky team with awesome goaltending. Spoiler alert: The Ducks win a one-goal game.
Entertainment quotient: 7/10 — With eight games, there isn’t a busier Sunday this season.
Monday, March 30 — Six games
The can’t-miss matchup: Kings at Blackhawks — One year after these teams played one of the greatest playoff series of all time in last year’s Western Conference final, they face each other with the Kings’ playoff hopes on the line.
Other good options: Lightning at Canadiens (they met in the playoffs last season and could be headed for an eventual rematch); Canucks at Blues (two good teams, and also a big stop on the Ryan Miller Regret Tour); Sabres at Coyotes (I’m really not kidding, I’m legitimately fascinated by how these games will play out).
No thanks: Oilers at Avalanche — I bet the disappointing team with all of those high draft picks that’s being run by former players with Cup rings ends up winning.
Entertainment quotient: 6/10 — A solid night of entertainment.
Tuesday, March 31 — Seven games
The can’t-miss matchup: Panthers at Bruins — This is the first of two remaining games that Florida has with Boston, and if Florida is going to have any chance of getting back in the race, it pretty much needs to win both. Roberto Luongo does great in big games in Boston, right?
Other good options: Senators at Red Wings (as Ottawa plays with one eye on the out-of-town scoreboard); Rangers at Jets (some interconference fun); Canucks at Predators (two Western playoff teams still fighting for seeding).
No thanks: Devils at Blue Jackets — Bad teams + great goalies = nope.
Entertainment quotient: 5/10 — Not great, but still not bad. And you’ll want to take what you can get, because tomorrow is awful.
Wednesday, April 1 — Four games
The can’t-miss matchup: Flyers at Penguins — Pretty slim pickings tonight, but at least these teams hate each other.
Other good options: Oilers at Ducks (if we’re stretching the definition of “good”); Avalanche at Sharks (if we’re really stretching the definition of “good”).
No thanks: Maple Leafs at Sabres — No. Just, no.
Entertainment quotient: 1/10 — Hi, it’s the NHL, and we made sure there were lots of good games tonight. April Fool’s!
Thursday, April 2 — Nine games
The can’t-miss matchup: Rangers at Wild — OK, this might be a weird pick, but man, this could be a fun matchup. These are basically the two hottest teams since the new year, with excellent goaltending and plenty of big-name stars. I’m not going to say the words “potential Stanley Cup preview,” because that would be crazy. But I’m also not going to not say them.
Other good options: Canucks at Blackhawks (we’re not that far removed from when this was the best rivalry in the league); Flames at Blues (a real tough test for a ridiculously likable Calgary team); Bruins at Red Wings (Detroit gets a chance to crush the playoff hopes of the team that eliminated them last year); Lightning at Senators (hey, remember when the Senators had Ben Bishop and basically gave him away? [Gets pelted with hamburgers.]).
No thanks: Hurricanes at Panthers — Do you miss the magic of the Southeast Division? Yeah, neither does anybody else.
Entertainment quotient: 7/10 — Did you enjoy your Wednesday night off? We’re into the stretch run.
Friday, April 3 — Five games
The can’t-miss matchup: Coyotes at Sharks — Only because I’m penciling this in as the game that officially eliminates the Sharks, and we can all enjoy the irony of the Sharks’ fake rebuild being wiped out by an actual rebuild. Also, the other games are pretty bad. Mostly that, actually.
Other good options: Avalanche at Ducks (in a battle of division leaders … from last year); Blues at Stars (St. Louis will probably need to bank all the points it can get to win the Central); Canadiens at Devils (although the Habs play the night before at home, so Carey Price probably sits out).
No thanks: Blackhawks at Sabres — I’ll just leave this here.
Entertainment quotient: 4/10 — One thing you learn from doing this feature year after year: The NHL really isn’t a Friday night sort of league.
Saturday, April 4 — 13 games
The can’t-miss matchup: Canucks at Jets — Two Canadian teams face each other in a game that should have playoff implications for both. Yes, I realize that’s not actually all that exciting, but this full slate of games is oddly uninspiring.
Other good options: Red Wings at Wild (a battle of former division rivals); Flames at Oilers (this rivalry usually serves up something worth watching); Capitals at Senators (for the wild-card implications); Avalanche at Kings (the champs continue to fight for their playoff lives).
No thanks: Flyers at Hurricanes — This is almost by default; it’s the night’s only game in which both teams will definitely be out of the playoff race.
Entertainment quotient: 7/10 — A weird night. Plenty of games, and yet nothing that really stands out as must-see. Get fresh batteries in the remote and you should be OK.
Sunday, April 5 — Five games
The can’t-miss matchup: Blues at Blackhawks — These are the two best teams in the Central, and this could be a showdown for first place. Even if it’s not, count on an old-fashioned Norris battle between two teams that expect to get to the conference final and know they have to go through each other to do it.
Other good options: Penguins at Flyers (again, probably not meaningful, but worth keeping an eye on for the bad blood); Canadiens at Panthers (assuming Florida is still hanging in the race, which is kind of a big if at this point); Capitals at Red Wings (two sneaky good Eastern playoff teams).
No thanks: Senators at Maple Leafs — This one will have a Battle of Ontario playoff feel, in the sense that we already know who’s going to win.
Entertainment quotient: 5/10 — The last Sunday of the regular season is … well, it’s OK. That’s about it.
Monday, April 6 — Five games
The can’t-miss matchup: Kings at Canucks — If the Kings are struggling, this could be a must-win, and a chance for the Canucks to go a long way toward finishing them once and for all. If L.A. is surging, this could be a first-round preview.
Other good options: Jets at Wild (a battle between the West’s two current wild-card teams); Blue Jackets at Rangers (Rick Nash something something); Hurricanes at Sabres (mainly because by this point, you’re going to be in net for Buffalo).
No thanks: Stars at Sharks — Here are two teams that were supposed to be good but weren’t, playing out the string and hating life.
Entertainment quotient: 5/10 — Not a great slate, although that Kings/Canucks game could be the sort of matchup that East Coast people will pretend to have stayed up to watch.
Tuesday, April 7 — Nine games
The can’t-miss matchup: Penguins at Senators — Sidney Crosby, gunning for the Art Ross, takes on Andrew Hammond, trying to drag the Senators to an unlikely playoff berth.9
Other good options: Coyotes at Flames and Kings at Oilers (two cases of a critical playoff matchup and serious tanking implications all rolled into one game); Wild at Blackhawks (Minnesota could be in range to catch the Hawks, and if not, it’s still a rivalry game and dark-horse playoff matchup).
No thanks: Hurricanes at Red Wings — Carolina is playing out the string, and the Red Wings seem locked into third in the Atlantic. But hey, it’s a rematch of that Stanley Cup final you have absolutely no recollection of.
Entertainment quotient: 6/10 — Nothing incredible, but with five nights left on the schedule, there should be something crucial going on somewhere.
Wednesday, April 8 — Three games
The can’t-miss matchup: Bruins at Capitals — Boston figures to still be hanging in the wild-card race. Washington could be, too, although it’s been playing well enough lately that it could move up in the Metro instead. Either way, this one should matter.
Other good options: Stars at Ducks (two entertaining teams meet, although almost certainly without any playoff implications).
No thanks: Leafs at Blue Jackets — It will be fun when the Columbus cannon fires and nobody on the Leafs bench even flinches, because they’ve all been dead inside since January.
Entertainment quotient: 2/10 — It’s Rivalry Wednesday! (In this case, the rivalry is between you and falling asleep 10 minutes in.)
Thursday, April 9 — 11 games
The can’t-miss matchup: Kings at Flames — Depending on how the Western wild-card race is shaking out, this battle for third in the Pacific could also be a must-win for both teams’ playoff hopes.
Other good options: Blackhawks at Blues (a rematch from four days prior, which is about as close we get these days to an old-fashioned home-and-home); Red Wings at Canadiens (a possible first-round preview between teams that haven’t met in the playoffs since 1978).
No thanks: Hurricanes at Flyers — I just searched YouTube to see if Ron Hextall ever attacked Ron Francis. No such luck.
Entertainment quotient: 8/10 — At this point, we’re stuck making plenty of assumptions about how the next two weeks play out. But that Kings/Flames game could be dynamite.
Friday, April 10 — Two games
The can’t-miss matchup: Islanders at Penguins — This one is looking like a possible first-round matchup, and this game could determine who gets home ice. And of course, these two teams don’t like each other very much.
Other good options: None. Literally none. It’s the penultimate night of the season, but there are only two games.
No thanks: Sabres at Blue Jackets — The only person watching this game will be Connor McDavid, who’ll have it on in the background as he sadly tries to figure out how many parkas he can fit into a suitcase.
Entertainment quotient: 1/10 — This is just awful. But that’s OK, because it’s the league’s way of setting us up for …
Saturday, April 11 — 15 games
The can’t-miss matchup: Flames at Jets — It’s hard to tell which games will really matter two weeks from now, but this one sure looks like it could decide the West’s last playoff spot. If so, it will be the biggest Jets/Flames game since the Dale Hawerchuk days. Also, the MTS Centre will be insane.
Other good options: Sharks at Kings (L.A. could come in needing a win to make the playoffs, setting up a nice payback scenario for San Jose); Rangers at Capitals (a possible first-round preview); Wild at Blues (another possible first-round preview); Bruins at Lightning (a tough final game for the Bruins, if they’re even still in the hunt); Habs at Leafs (no doubt the Toronto crowd will give their beloved Leafs quite the send-off).
No thanks: Devils at Panthers — I’m assuming the Panthers are out by now. If so, there’s really no reason to watch, other than seeing if Jaromir Jagr gets confused over which team he plays for.
Entertainment quotient: 10/10 — Now that’s how you close out a season. With all 30 teams in action over 12 hours, sheer volume pretty much guarantees that we’ll get at least a couple of critical matchups. It should all add up to more than enough to make up for having the next few days off.

Revisits And Continuations
Lots of things getting back into the swing today. Good times, good times.
- First of all, I don’t wish to get up any hopes, but it appears that there is a new content page at Broodhollow¹, following a chapter title page last week. It’s been a long hiatus, what with Kris Straub welcoming a small alive human into his life and designing the book 2 Kickstarter and in his copious free time making all kinds of other media.
There’s a million ideas inside of Straub, all demanding to be brought into the world in some form or another, but I suspect that Broodhollow is the one that he will look back on as the most personal and closest to his heart … and the jokes on the new page ain’t bad either. Welcome back to West Virginia’s most eccentric town; hope you decide to set and stay a while.
- Likewise, I don’t want anybody getting too excited, but in the past week we’ve seen as many updates to the long-paused Misery Loves Sherman as we’ve seen in the past four years. I know that Chris Eliopoulos has been busy, what with lettering every other comic book on the stands, as well as writing and illustrating them². Creating a webcomic takes time and he’s a man with little enough of it, but it’d be good to see Sherman & company back on the regular.
- Sort of a relaunch, sort of not: Samantha Leriche-Gionet, known professionally as Boum, has been chronicling her life at Boumeries for more than four years now (if you haven’t read it, it’ll make you think of American Elf), and three years back she did a print graphic novel, La Petite Révolution. Starting today, she’s serializing Révolution online in the original French and also translated for the first time into English; A Small Revolution will update Tuesdays and Thursdays, with five pages up today to get you started.
The main character is young, a street-dwelling orphan, and deceptively cute. I suspect that she is going to surprise everybody — in both the uprising and the oppressive dictatorship — with her determination to make things change, and woe betide those that underestimate her. Never forget: revolutions are where the old scores get settled. For me, I think I’ll read the English and French pages side-by-side, see how much I can make out from context. I’m a long way from studying French in high school³, but there are so many good comics in French, brushing up can only be a good thing.
Spam of the day:
Hello to every , because I am in fact keen of reading this blog’s post to be updated on a regular basis. It carries fastidious data.
I have achieved the pinnacle of hack webcomics pseudojournalizing. Did Eric Burns-White ever get complimented for the fastidiousness of his data? I think not. Suck it, every other webcomics blog!
________________
¹ That one menu tells you more about the town and the place/time it occupies than any three pages of text could. Bravo.
² Not to mention dealing with what must be more-than-infrequent confusion over the fact that there is another Chris Eliopoulos working in comics. I mean, there are other Gary Tyrrells in the world, but none of them are in the field of hack webcomics pseudojournalism.
³ Although, more than 20 years after studying or speaking it, I retained enough to conduct Eurailpass redemptions with a ticket agent in Brussels, and also to achieve my basic standard for functionality in a language: I was able to obtain a room for the night, a meal, and a beer.
Hark, A Vagrant: Kokoro Pt 1
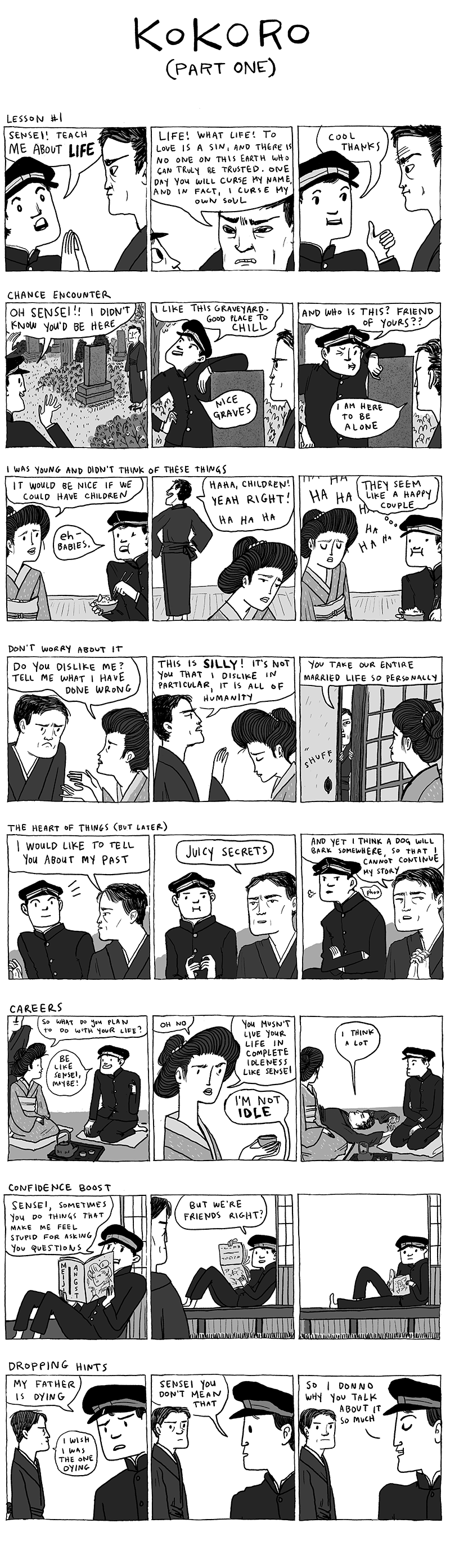


buy this print!
Kokoro is one of those books that maybe you have to read in a class, and you think that it's kind of dry, but then somehow it gets under your skin and you can't stop thinking about it. And I guess they call them classics because they stick around like that.
The Rise of Nintendo: A Story in 8 Bits
The following is an excerpt from Blake J. Harris’s new book, Console Wars. It has been slightly modified for this publication.
On September 23, 1889, just weeks before his thirtieth birthday, an entrepreneur named Fusajiro Yamauchi opened a small, rickety-looking shop in the heart of Kyoto. To attract the attention of passing rickshaws and wealthy denizens, he inscribed the name of his new enterprise on the storefront window: Nintendo, which had been selected by combining the kanji characters nin, ten, and do. Taken together, they meant roughly “leave luck to heaven” — though, like most successful entrepreneurs, Yamauchi found success by making his own luck. In an era where most businessmen were content to survive off the modest returns of regional mainstays such as sake, silk, and tea, he decided it was time to try something new. So instead of selling a conventional product, Fusajiro Yamauchi opted for a controversial one, a product that the Japanese government had legalized only five years earlier: playing cards.
In the heart of Kyoto, he and a small team of employees crafted paper from the bark of mulberry trees, mixed with soft clay, and then added the hanafuda card designs with inks made from berries and flower petals. Nintendo’s cards, particularly a series called Daitoryo (which featured an outline of Napoleon Bonaparte on the package), became the most successful in all of Kyoto.
After several decades of staggering success, Fusajiro Yamauchi retired in 1929 and was succeeded by his son-in-law Sekiryo Yamauchi, who ran Nintendo efficiently for nineteen years, but in 1948 he had a stroke and was forced to retire. With no male children, he offered Nintendo’s presidency to his grandson, Hiroshi, who was twenty-one and studying law at Waseda University. It didn’t take long for Hiroshi Yamauchi to make his presence known. He fired every manager that had been appointed by his grandfather and replaced them with young go-getters who he believed could usher Nintendo beyond its conservative past.
With innovation on his mind,Yamauchi branched out into a number of other, less lucrative endeavors, including an instant-rice company and a pay-by-the-hour “love hotel.” These disappointments led Yamauchi to the conclusion that Nintendo’s greatest asset was the meticulous distribution system that it had built over decades of selling playing cards. With such an intricate and expansive pipeline already in place, he narrowed his entrepreneurial scope to products that could be sold in toy and department stores and settled upon a new category called “videogames.”
Yamauchi wanted Nintendo to aggressively get into the videogame business, which was really two separate businesses: home consoles and coin-operated arcade games. He saw the potential in these industries and took the necessary steps for Nintendo to enter both. Despite middling results from titles like Wild Gunman and Battle Shark, Yamauchi remained committed to his new vision and continued to allocate a vast amount of resources toward videogames. In 1977 Nintendo released a shoebox-sized orange console called the Color TV-Game 6, which played six slightly different versions of electronic tennis and was met with a mixed reception. Though the console managed to sell one million units, it ultimately lost money. Nevertheless, Yamauchi remained undeterred. Nintendo continued to put out arcade games (striking out with duds like Monkey Magic and Block Fever) and also continued to release home consoles (like the Color TV-Game 15, which offered fifteen slightly different versions of electronic tennis).
By this point, Nintendo already had penetrated most of Japan, and so Yamauchi set his sights on the place where the videogame frenzy had started: America.
1. Arakawa
Yamauchi had dipped a toe into this red, white, and blue pool a few years earlier and was encouraged by the results. In the late 1970s Nintendo had begun working with a trading company that would export arcade cabinets to American distributors, who would in turn sell these games to vendors in the United States. Though the profits from this arrangement were minimal, Yamauchi believed that if he could cut out the trading companies and send over someone he trusted to grow Nintendo’s business organically, then there was a lot of money to be made.
The American market would be risky, tricky, and perpetually persnickety. There appeared to be only one man properly equipped for the challenge: Minoru Arakawa, a frustratingly shy but brilliant thirty-four-year-old MIT graduate. Not only did Arakawa possess the insight and intellect to open a U.S. division of Nintendo, but he was already living in North America and had an incalculable fondness for America (some of his most cherished memories had come from a post college cross-country trip he’d taken in a used Volkswagen bus).
In every way, Minoru Arakawa appeared to be the perfect candidate … except that he happened to be married to Yamauchi’s daughter, Yoko, who blamed Nintendo for turning her father callous. She simply refused to let her husband join Nintendo, as she did not want to watch history repeat itself.
Yamauchi initially proposed the idea to Arakawa in early 1980. Following a pleasant family dinner, Yamauchi spent two hours discussing his plans for the expansion of Nintendo and concluded by stating that the success of his plan hinged on Arakawa. Anticipating his daughter’s reluctance, Yamauchi explained that this American division would be a completely independent subsidiary. Arakawa wrestled with the decision as well as with the objections of his wife, who cautioned him that no matter what he accomplished, he would always be perceived as nothing more than the son-in-law. Arakawa decided that the opportunity was too good to pass up, and in May 1980 he and his wife left Vancouver to start Nintendo of America (NOA).
Arakawa and his wife spent their days at the office and their nights observing games and players at local arcades. They learned a lot this way, but no amount of knowledge could make up for the fact that for Nintendo to gain a foothold, they needed a strong sales network. So Arakawa set up a meeting with a couple of guys who he thought might be able to help: Al Stone and Ron Judy.
2. Stone and Judy
Al Stone and Ron Judy were old friends from the University of Washington, where they had lived in the same frat house and had been known to embark on promising get-rich-quick schemes together (like buying soon-to-be-discarded local wine cheaply and then reselling it to their college brethren with less sophisticated palates). After graduating, they started a trucking business in Seattle called Chase Express. Chase struggled, and while they continued to invest in it and hope for a turnaround, they also began looking for alternative business opportunities.
They eventually found their answer, though it still involved big rigs. Through a friend in Hawaii, Ron Judy had been informed that a Japanese trading company was seeking a distributor to sell some arcade games made by Nintendo. Intrigued, he agreed to test the waters and received a crate containing a few arcade cabinets of Nintendo’s Space Fever. Though the game was little more than a shameless rip-off of Taito’s Space Invaders, Judy got his brother-in-law to place the games in some of the taverns he owned in south Seattle. Much to his delight, the machines were quickly overrun with quarters, which convinced him and Stone that this was their future. They formed a distribution company called Far East Video and used their assets from the trucking industry to travel around the country and sell Nintendo games to bars, arcades, hotel lounges, and pizza parlors.
Space Fever was followed by Space Launcher (underwhelming), which was followed by Space Firebird (disappointing), which was followed by a slew of unsuccessful non-space-themed games. After this string of mediocre misfires, Stone and Judy were ready to quit, and Arakawa couldn’t help but reconsider his new vocation. More than ever, Nintendo of America needed a megahit, like Pong or Pac-Man. And just when it appeared time was running out, Arakawa believed that he’d found what he needed: Radarscope.
At first glance, Radarscope may have appeared to be just another shoot-’em-up space game, but it distinguished itself with incredibly sharp graphics and an innovative 3-D perspective. After receiving positive feedback from test locations around the Seattle area, Arakawa invested much of NOA’s remaining resources in three thousand units. But a few weeks later, before the rest of the arcade cabinets even arrived, Arakawa felt an ominous chill upon revisiting the test locations, where he noticed that nobody was playing his crucial new game. That foreboding was validated after the three thousand units finally arrived and Stone and Judy found that operators had little interest. Radarscope was fun at first, the consensus appeared to be, but it lacked replay value.
3. Miyamoto
With so much invested in this game, the last remaining hope was for a designer in Japan to quickly create a game and send over processors with that new game to America, where NOA employees could swap out the motherboard and then repaint the arcade cabinets. This task was given to Shigeru Miyamoto, a floppy-haired first-time designer who believed that videogames should be treated with the same respect given to books, movies, and television shows. His efforts to elevate the art form were given a boost when he was informed that Nintendo was close to finalizing a licensing deal with King Features, enabling him to develop his game around the popular cartoon series Popeye the Sailor Man. Using those characters, he began crafting a game where Popeye must rescue his beloved Olive Oyl by hopping over obstacles tossed in his way by his obese archenemy, Bluto.
Shipments containing the code for Miyamoto’s new game began to arrive. Due to last-minute negotiation issues with King Features, Nintendo had lost the rights to Popeye, which forced Miyamoto to come up with something else. As a result, Arakawa, Stone, Judy, and a handful of warehouse employees didn’t know what to expect. They inserted the new processor into one of the thousands of unsold Radarscope machines and then watched the lights flicker as the words “Donkey Kong” came to life on the arcade screen. The initial impression was that this was a silly game with an even sillier name. Who would possibly want to play a game where a tiny red plumber must rescue his beloved princess by hopping over obstacles tossed in his way by an obese gorilla? Yet, with no remaining options, Stone and Judy set out across the country to sell it.
Never before had there been a quarter magnet quite like Donkey Kong. It was so successful, in fact, that it eventually attracted the attention of a major Hollywood studio, whose high-priced legal team believed that the game violated copyrights, and they threatened to crush Nintendo. To avoid this potentially crippling blow, Arakawa turned to the only lawyer he knew in Seattle: Howard Lincoln, an elegant, imposing former naval attorney whose only claim to fame was having modeled for Norman Rockwell’s painting The Scoutmaster when he was a child.
4. Lincoln
Lincoln had first crossed paths with Arakawa about one year earlier when Stone and Judy needed him to review their contract with Nintendo of America. After that, Lincoln slowly but surely took on the role of Arakawa’s consigliere, weighing in on any matter with legal ramifications. As Nintendo of America grew, Lincoln drew up new employment agreements, looked at various business deals, and handled some tough matters (like siccing the U.S. marshals on Donkey Kong counterfeiters). Through it all, Lincoln and Arakawa forged an unshakable friendship. Which is why Lincoln was the first person Arakawa contacted when, in April 1982, MCA Universal sent a telex to NCL explaining that Nintendo had forty-eight hours to hand over all profits from Donkey Kong due to the game’s copyright infringement on their 1933 classic King Kong.
It didn’t take long for them to realize that this was a high-stakes shakedown. Though never explicit, Universal’s ultimatum was simple: Settle or we’ll make life at Nintendo so difficult that the company will fold. The prudent thing to do was pay the ransom. But Lincoln had an ace tucked up his sleeve: in all of his research, there didn’t appear to be a single document indicating that Universal had trademarked King Kong, which would place the gorilla in the public domain. And in early 1983, Judge Robert W. Sweet sided with Nintendo. He concluded that they had not infringed and, as Lincoln had predicted earlier, he awarded Nintendo over $1 million in legal fees and damages.
The Donkey Kong fiasco-turned-feat caused many ripples, but three waves in particular were instrumental in creating the eventual tsunami that would be Nintendo. First, Lincoln became NOA’s senior vice president. Second the countersuit set the tone for the litigious stance that many would say later defined the company. And third — and most importantly — the verdict kept the Donkey cash flowing, providing Nintendo with a war chest at what would soon prove to be a crucial moment.
5. Borofsky and Associates
By the early 1980s, the videogame bonanza had become so lucrative that everyone wanted in. This included companies that had no business entering the market (like Purina), companies that didn’t understand the market (like Dunhill Electronics, whose Tax Avoiders allowed players to jockey past a maze of evil accountants and onerous IRS agents), and lowbrow outfits that polarized the market (like Mystique, whose flair for pornographic titles was highlighted by their 1982 anticlassic Custer’s Revenge, which follows a naked cowboy on his quest to rape Native American women). The marketplace was overrun by a glut of smut, muck, and mediocrity.
And just like that, the North American videogame industry ground to a halt. Hardware companies (like Atari) went bankrupt, software companies (like Sega) were sold for pennies on the dollar, and retailers (like Sears) vowed never to go into the business again. Meanwhile, Nintendo quietly glided through the bloody waters on a gorilla-shaped raft. The continuing cash flow from Donkey Kong enabled Arakawa, Stone, Judy, and Lincoln to dream of a new world order, one where NOA miraculously resurrected the industry and Nintendo reigned supreme. Not now, perhaps, but one day soon.
In Japan, however, that time had already come. Yamauchi’s large investment in R&D had paid off in the Family Computer. The Famicom, as it was commonly called, was an 8-bit console that stood head and shoulders above anything that had ever come before. It was released in July 1983 along with three games: Donkey Kong, Donkey Kong Jr., and Popeye, which Miyamoto ended up designing after licensing negotiations got back on track. The Famicom stumbled out of the gate but was soon rescued by heavy advertising and the release that September of Super Mario Bros. (another Miyamoto brainchild).
As sales soared to staggering heights, Yamauchi pressured his son-in-law to introduce the Famicom in America. Arakawa resisted, exercising patience. The U.S. market was still licking its wounds from the videogame crash, and releasing the right console at the wrong time would be a recipe for disaster. For this reason, he continued to rebuff the suggestion until 1984, when he was finally willing to consider the notion— but only if the console Nintendo of America sold looked nothing like a console at all.
This wolf-in-sheep’s-clothing logic led to the Advanced Video System (AVS). Though the guts of this machine were nearly identical to the Famicom’s, the AVS hardly resembled its foreign relative. It came with a computer keyboard, a musical keyboard, and a cassette recorder; and aesthetically, it was slim and sleek, with a subdued gray coloring that contrasted sharply with the Famicom’s peppy red and white palette. Nintendo’s AVS, the non-console console, was first introduced at the 1984 Winter Consumer Electronics Show. Arakawa simply wanted to gauge the market reaction, which was distressing: nothing but scoffs, sighs, and sob stories. Nobody there wanted anything to do with the AVS, except for a tanned man with piercing blue eyes who stared at the Advanced Video System as if it were the sword in the stone. He then introduced himself with an understated sureness that would have made even King Arthur jealous. His name was Sam Borofsky.
Borofsky ran Sam Borofsky Associates, a marketing and sales representative firm based in Manhattan. Back in the late seventies, they became one of the first firms to represent videogames, and at the height of the boom they had been responsible for over 30 percent of Atari’s sales. If Nintendo of America ever wanted retailers to reopen their doors, then these were the guys who ought to do the knocking. From Borofsky’s end, the attraction was equally strong. Ever since Atari had imploded, he’d been scouring the country in search of the next big thing, and as he reviewed what Nintendo had to offer, he believed he had found it.
Arakawa, however, still needed convincing, which Borofsky was happy to provide. He spent months detailing the reasons for Atari’s downfall and outlining plans for a proposed launch. Meanwhile, Nintendo of America put another new costume on the Famicom, dressing it up as an all-in-one entertainment center for kids. The result of the rebranding effort was a clunky gray lunchbox-like contraption and, along with that, a new lexicon to differentiate it from its predecessors: cartridges were now dubbed Game Paks, the hardware was dubbed the Control Deck, and the entire videogame console was rechristened the Nintendo Entertainment System (NES). And to round out the renovation, the NES came with a pair of groundbreaking peripherals: a slick light-zapper gun and an amiable interactive robot named R.O.B.
6. The Launch
Focus groups suggested that the NES would be a colossal flop, R.O.B. kept malfunctioning during pitches, and the press showed no interest. Arakawa, however, remained undeterred. He temporarily relocated a handful of employees to the East Coast after leasing a warehouse in Hackensack, New Jersey, where Nintendo could house inventory, build in-store displays, and, most important, resemble a legitimate company to still-skeptical retailers. To keep tabs on the progress, Ron Judy made frequent visits to New York, often accompanied by Bruce Lowry, NOA’s bright and blustery VP of sales. Lowry was occasionally able to help Borofsky persuade the big toy chains. At the top of their wish list was Toys “R” Us, whose eventual decision to stock the NES provided Nintendo with much-needed momentum going into the launch.
On the morning of the big day, the Nintendo of America team gathered at FAO Schwarz, where Nintendo had paid for an elaborate window display and an attractive floor space that featured a small mountain of televisions with game footage playing. The moment of truth had finally arrived, and within moments of the store’s opening an excited customer eagerly approached the display, grabbing an NES and all fifteen of its games. The NOA team looked on, watching everything they had been working toward so suddenly come to fruition. It was a dream come true — until they were snapped back to reality upon learning that Customer #1 was actually just a competitor doing due diligence.
That Christmas, the NES was available in over five hundred stores. Though no staggering success, Nintendo managed to sell half of the 100,000 units they’d stocked, which effectively proved to the world that the videogame industry was not dead but had simply been hibernating.
Heading into 1987, what NOA really needed was someone to help roll out the NES nationwide and ensure that at the end of this roller-coaster ride, Nintendo would wind up on top. Someone to prove that the NES was more than just this year’s Christmas fad. Someone who could exploit the potential for expansion and transform Nintendo from a niche sensation into a global juggernaut.
7. Main
That someone turned out to be Peter Main, though at the time he was dealing with matters much more pressing than corporate expansion: beef dip sandwiches and garlic butter buns. As the president of White Spot, a Canadian fast-food chain, Main was used to eating, sleeping, and breathing burgers, but in the summer of 1985 his mind went into overdrive when an outbreak of botulism swept through Vancouver. Health officials alleged that improper refrigeration of garlic oil concentrate was the likely cause and that Main’s restaurants were responsible for the epidemic. Following this horrifying news, he spent much of the next year doing damage control, ensuring that the issue had been resolved and defending the integrity of his beef dip sandwich. When the public outcry finally died down and White Spot’s reputation was restored, he stepped down from his post and took a long vacation to decide what he’d like to do next. That’s when Arakawa called and asked Main to join Nintendo of America.
Before Peter Main and Minoru Arakawa were ever colleagues they were friends, and ever since Arakawa had left Vancouver to start NOA, he had been trying to recruit Main. For years Main declined these job offers. Videogames were a far cry from hamburgers, but even though he turned down Arakawa, he often provided friendly advice on Nintendo of America’s strange yet profitable forays into the restaurant business (Arakawa had gone ahead and bought the British Columbia franchise rights to Chuck E. Cheese as well as a pair of seafood bistros in Vancouver). Main’s expertise as a restauranteur only fueled Arakawa’s desire to rope him in, but time after time Main declined the overtures — until that fateful call in late 1986. This time Main was open to a major life change, and it didn’t hurt that Ron Judy was planning to relocate to Europe, which would effectively make Main NOA’s number three.
Though Main lacked any videogame experience, his outsider mentality allowed him to look at the business as something novel and spectacular. To spread this new gospel, he choreographed what he would later describe as Nintendo’s “storming of Normandy,” a full-out advertising, promotion, and distribution blitz that accompanied the rollout of the NES into stores nationwide. Meanwhile, Main provided a trustworthy-looking corporate (and Caucasian) face to a company that many in the outside world still viewed as a foreign curiosity. Main was an expert charmer. And that charm, that talent for cultivating friendships, gained the company credibility with Wall Street, trust from retailers, and respect from parents wanting to know what they were buying.
Month after month, Nintendo of America grew stronger. They sold 2.3 million consoles in 1987 and 6.1 million in 1988. As staggering as these numbers were, sales of the hardware were nothing compared to the software: the company unloaded 10 million games in 1987, and 33 million more in 1988. With numbers like these, it didn’t take Main long to realize that, at the end of the day, the console was just the movie theater, but it was the movies that kept people coming back for more. This personal revelation led to a Hollywood-like title-driven business strategy, and his coining of the phrase “the name of the game is the game.”
Main’s approach to sales and marketing coincided with Arakawa’s overarching philosophy of “quality over quantity.” As Nintendo exploded, there were plenty of opportunities to make a quick buck (hardware upgrades, unnecessary peripherals), exploit the company’s beloved characters (movies, theme parks), or dilute the brand by trying to attract an audience older than Nintendo’s six-to-fourteen-year-olds. But these kinds of things didn’t interest Arakawa. What propelled him was a desire to continually provide Nintendo’s customers with a unique user experience. He set up a toll-free telephone line where Nintendo “Game Counselors” were available all day to help players get through difficult levels, and he initiated the Nintendo Fun Club, which sent a free newsletter to any customer who had sent in a warranty card. Both programs were very costly and could have been offset by charging small fees or obtaining sponsorship, but Arakawa believed that doing so would compromise Nintendo’s mission. And to further safeguard Nintendo from the dangers of impurity, he and his team put into place a series of controversial measures:
1. The Nintendo Seal of Quality: Ron Judy had the novel idea of mandating that all games pass a stringent series of tests to be deemed Nintendo-worthy, ensuring high-caliber product and making software developers beholden to Nintendo’s approval.
2. Third-party licensing program: Howard Lincoln’s strict licensing agreement enabled software designers to make games for the NES but restricted the quantity they could make (five titles per year), required full payment up front (months before revenue from a game would be seen), and charged a hefty royalty (around 10 percent). In addition to these stringent terms, all game makers needed to purchase their cartridges directly from Nintendo.
3. Inventory management: Heeding Sam Borofsky’s suggestion, Peter Main devised an incredibly rigid distribution strategy that purposefully provided licensees and retailers with only a fraction of the products they requested. The goal of this technique was twofold: to create a frenzy for whatever products were available, and to protect overeager industry players from themselves.
Though NOA’s methods drew ire from retailers, anger from software developers, and eventually allegations of antitrust violations from the U.S. government, there was no denying that whatever Nintendo was doing was working — so well, in fact, that Peter Main needed additional reinforcements as he inflicted Nintendo-mania on his adopted homeland.
8. Nintendo Power
Help came in the form of Bill White, a straitlaced marketing whiz whose smallish eyes and oversized, round-rimmed glasses emitted a boyish vibe. Though he was only thirty years old (and, after a haircut, could have passed for thirteen), he spoke about brand recognition, market analysis, and strategic alliances with the expertise of someone twice his age. Part of that precocious nature was due to an almost religious belief in the power of marketing, part was due to his father’s history as a Madison Avenue ad man, and part was due to a chronic insecurity that could only be quieted by winning at everything he did. Peter Main saw the potential in White and hired him in April 1988 to become Nintendo’s first director of advertising and public relations.
When White joined NOA, the marketing department consisted of just three people: himself, Main, and Gail Tilden, an exceptionally smart woman with an encyclopedic memory. The lack of manpower forced White to wear many hats, but his most important responsibility was to forge corporate partnerships. Though Nintendo continued the take the videogame world by storm, the rest of the world still didn’t know what a Nintendo was. To build the brand, White courted Fortune 500 companies, resulting in pivotal promotions, like Pepsi placing a Nintendo ad on over 2 billion cans of soda and Tide featuring Mario on the detergent maker’s giant in-store displays. His biggest coup came with the release of Super Mario 3, when he negotiated for McDonald’s to not only make a Mario-themed Happy Meal but also produce a series of commercials centered around the game. By virtue of his efforts, White became Main’s right-hand man, something of a protégé. But as Main fed White’s ambitions and the young marketer swallowed up more and more responsibility, this left Tilden, the other member of the marketing team, with less and less to do. This displeased Arakawa, who set out to find a better way to utilize one of NOA’s most dynamic employees.
Tilden was at home, nursing her six-week-old son, when Arakawa called and asked her to come into the office the next day for an important meeting. So the following day, after dropping off her son with some trusted coworkers, she went into a meeting with Arakawa. The appetite for Nintendo tips, hints, and supplemental information was insatiable, so Arakawa decided that a full-length magazine would be a better way to deliver exactly what his players wanted.
Tilden was put in charge of bringing this idea to life. She didn’t know much about creating, launching, and distributing a magazine, but, as with everything that had come before, she would figure it out. What she was unlikely to figure out, however, was how to become an inside-and-out expert on Nintendo’s games. She played, yes, but she couldn’t close her eyes and tell you which bush to burn in The Legend of Zelda or King Hippo’s fatal flaw in Mike Tyson’s Punch-Out!! For that kind of intel, there was no one better than Nintendo’s resident expert gamer, Howard Phillips, an always-smiling, freckle-faced videogame prodigy.
Technically, Phillips was NOA’s warehouse manager, but along the way he revealed a preternatural talent for playing, testing, and evaluating games. After earning Arakawa’s trust as a tastemaker, he would scour the arcade scene and write detailed assessments that would go to Japan. Sometimes his advice was implemented, sometimes it was ignored, but in the best-case scenarios he would find something hot, such as the 1982 hit Joust, alert Japan’s R&D to it, and watch it result in a similar Nintendo title — in this case a 1983 Joust-like game called Mario Bros. As Nintendo grew, Phillips’s ill-defined role continued to expand, though he continued to remain the warehouse manager. That all changed, however, when he was selected to be the lieutenant for Tilden’s new endeavor.
In July 1988, Nintendo of America shipped out the first issue of Nintendo Power to the 3.4 million members of the Nintendo Fun Club. Over 30 percent of the recipients immediately bought an annual subscription, marking the fastest that a magazine had ever reached one million paid subscribers. And as the magazine’s audience grew, so did the influence of Howard Phillips.
If Nintendo Power gave kids a chance to step inside the candy factory, then he was their Willy Wonka, magically and eccentrically showing them how the sweets were made. Though Mario was Nintendo’s mascot, Phillips became the face of Nintendo. Peter Main took advantage of this, sending Phillips all over the country for press events and on-camera interviews. Main appointed Phillips Nintendo’s first Game Master. Shortly thereafter, Howard Phillips became a national celebrity, boasting a Q score higher than Madonna, Pee-wee Herman, and the Incredible Hulk.
The rise of the Game Master was just the latest sign of Nintendo’s unprecedented success. By 1990, Nintendo of America had sold nearly thirty million consoles, resulting in an NES in one out of every three homes. Videogames were now a $5 billion industry, and Nintendo owned at least 90 percent of that. The numbers were astounding, but Nintendo’s triumph went beyond that. Arakawa had proven that he was more than just the son-in-law, Lincoln had proven that he could take on anyone, and Main had proven that he could swim with the sharks.
Together, they had single-handedly resurrected an industry. And they did it all with only 8 bits. Imagine what they could do with 16 …
Blake J. Harris is a writer and filmmaker based in New York. He is currently codirecting the documentary based on his book, which is being produced by Scott Rudin, Seth Rogen, and Evan Goldberg. He will also serve as an executive producer on Sony’s feature-film adaptation of Console Wars.
Illustration by Kickpixel.

Your Guide to the Rest of the NHL Regular Season
Today is April 1, which means two things: Any minute now, the Toronto Maple Leafs will shout “April Fools’!” and we’ll all have a good laugh about the series of increasingly dumb things they’ve spent the year saying and doing; and the NHL regular season is heading down the homestretch.
That last part is probably more important to the rest of you, so let’s focus on that. We’ve got less than two weeks of action left before the season concludes on April 13. With all four wild-card spots up for grabs and every team other than the Bruins and Penguins still fighting for playoff seeding, there are plenty of important nights left on the NHL regular-season calendar.
Of course, they can’t all be winners. So to help you plan your next few weeks of viewing, here’s a day-by-day breakdown of the April schedule.
Tuesday, April 1: 11 games
The can’t-miss matchup: Dallas at Washington — An interconference battle between two teams that are just on the outside of the playoff picture and desperate for points. (Why, yes, this will end up being a three-point game, why do you ask?)
Other good options: Montreal at Tampa Bay is a first-round playoff preview that will help decide who gets home ice. Rangers at Canucks gives Alain Vigneault a chance to all but bury the playoff hopes of the team that fired him. The Flames visit Toronto in Brian Burke’s first trip back to Toronto since he was fired last January, and if he doesn’t spend the whole game pelting Dave Nonis with waffles then I’ll never forgive him.
No thanks: There’s really no reason to watch Panthers at Islanders, unless you’re a top-five prospect in this year’s draft. By the way, the Islanders are going to show up a few times in this section. Sorry about that, Islander fans.
Entertainment quotient: 8/10 — This is shaping up as a fun start to the month.
Wednesday, April 2: Four games
The can’t-miss matchup: Boston at Detroit — These two Original Six teams haven’t met in the playoffs since 1957, but could be headed for a first-round matchup this year.
Other good options: The Coyotes can solidify their playoff spot with an upset win in Los Angeles. Oilers at Ducks is a Viktor Fasth homecoming, which isn’t really interesting, but there are only four games tonight so I’m reaching.
No thanks: The Senators and Islanders are a completely forgettable pairing.
Entertainment quotient: 2/10 — Pass.
Thursday, April 3: Nine games
The can’t-miss matchup: Los Angeles at San Jose — The Sharks need to keep winning to have any shot at the division title. If they don’t, then this is a preview of what’s likely to be the first round’s most brutal matchup.
Other good options: The Sabres visit the Blues for the first time since the Ryan Miller trade. Both the Wild at Blackhawks and Jackets at Flyers should will be key games for the wild-card races. Rangers at Avs features two good teams. Boston at Toronto is just a jerk move by the schedule maker at this point.
No thanks: I guess it’s Flames at Lightning by default.
Entertainment quotient: 7/10 — There’s something for everyone.
Friday, April 4: Seven games
The can’t-miss matchup: Capitals at Devils is probably a must-win for New Jersey, assuming it’s even still in the wild-card race.
Other good options: Canadiens at Senators has a good chance to be one of those “Ottawa’s building gets taken over by visiting fans” games, which are always fun. Blackhawks at Blue Jackets is a chance for Columbus to continue its playoff push with a huge win over its former quasi rivals.
No thanks: Literally nobody will watch Flames at Panthers.
Entertainment quotient: 4/10 — There are enough games that one or two should turn out to be good, but on paper this looks like kind of a dud.
Saturday, April 5: 11 games
The can’t-miss matchup: Colorado at St. Louis — Two of the Central’s powerhouse teams face off. The Avs are missing Matt Duchene and headed toward a first-round matchup with the Blackhawks that nobody will pick them to win. Beating the Blues in St. Louis could go a long way toward changing that perception.
Other good options: Flyers at Bruins is a rematch of a great game from Sunday, and has an outside shot at being a first-round preview. Kings at Canucks could be fun if Vancouver is still hanging around the playoff race. Wings at Habs should be a good one, and even if it isn’t, we’ll all have to pretend it is since it’s an Original Six matchup.
No thanks: Given the way San Jose seems to play down to the level of its competition, I wouldn’t recommend Predators at Sharks.
Entertainment quotient: 6/10 — It’s not a bad slate, but you’d think the league could do better on the penultimate Saturday night of the season.
Sunday, April 6: Six games
The can’t-miss matchup: Blues at Blackhawks — This is the nationally televised Sunday afternoon game, and it’s a good one. It could also be a Central final preview.
Other good options: The Penguins visit the Avalanche in a meeting of top 10 teams. The Stars visit the Panthers in what could be the most awkward chapter of the Tim Thomas/Roberto Luongo rivalry yet.
No thanks: The Blue Jackets host the … uh … Islanders. Sorry, guys, at least I had your back last year.
Entertainment quotient: 4/10 — Not much to get excited about, but that Blues/Hawks battle should be a must-see.
Monday, April 7: Three games
The can’t-miss matchup: Blues at Blackhawks — Seriously, just record Sunday’s game and rewatch it tonight.
Other good options: Flames at Devils? Nope. Wild at Jets? Don’t think so. Ducks at Canucks? Maybe, if we’re feeling especially kind, but no.
No thanks: See above. This entire day is a big “no thanks.”
Entertainment quotient: 1/10 — The NHL knows this is the last week of the season, right?
Tuesday, April 8: 10 games
The can’t-miss matchup: Coyotes at Blue Jackets — A lot can change in a week, but right now these look like two teams that will be desperately chasing wins as they try to secure wild-card spots.
Other good options: The Capitals face the Blues in a game that should have implications for both the Eastern wild-card race and the Presidents’ Trophy. Colorado at Edmonton probably won’t be good, but will at least give the two teams a chance to compare notes on their rebuilds.
No thanks: The Senators and Islanders are a completely forgettable pairing. We’ve used that line already, but it’s OK because you forgot.
Entertainment quotient: 5/10 — Lots of games and lots of possible playoff teams in action, but a shortage of really intriguing matchups.
Wednesday, April 9: Five games
The can’t-miss matchup: San Jose at Anaheim — This could end up being a showdown for the Pacific Division title. The winner gets to host a wild-card team, while the loser has to play the Kings. The stakes don’t get much higher in the regular season.
Other good options: Detroit at Pittsburgh will help determine whether the Wings can continue their playoff streak, and doubles as a possible first-round matchup. Montreal at Chicago features two teams fighting for home-ice advantage in Round 1. The Blue Jackets visit the Stars to make up the Rich Peverley game, which will be confusing because it will start with the score 1-0.
No thanks: L.A. at Calgary doesn’t have much going for it, unless the Flames give Darryl Sutter a “welcome back” scoreboard video that’s just a montage of him making Darryl Sutter faces.
Entertainment quotient: 9/10 — Almost all of it for that Sharks/Ducks showdown, which if we’re lucky could be the biggest game of the month.
Thursday, April 10: 11 games
The can’t-miss matchup: Toronto at Florida — The Maple Leafs look to continue their late-season playoff push! Or, in a slightly more likely scenario, the Maple Leafs try to snap their 12-game losing streak under the leadership of new head coach Riddick Bowe!
Other good options: Honestly, I have no idea. This is a weird day — it features a ton of playoff bubble teams, with Washington, Minnesota, Phoenix, and New Jersey all in action. Some of those games will be absolutely critical, but there’s no way of knowing which ones it will be 10 days from now, and none of the other matchups really stand out as must-see.
No thanks: The Jets, who have nothing to play for, host the Bruins, who’ll have the East’s top seed wrapped up and will probably be focused on just staying healthy.
Entertainment quotient: 7/10 — With 11 games on the schedule, some of them have to be good. We just don’t know which ones.
Friday, April 11: Seven games
The can’t-miss matchup: St. Louis at Dallas — Well, the Stars could be out of the race by then. But if not, they’ll be fighting for their playoff lives against a team looking to wrap up the Presidents’ Trophy. And it could be a first-round preview.
Other good options: The Caps host the Hawks and the Wings host the Hurricanes in a pair of games that could help decide the East’s wild-card picture. Colorado at San Jose will feature two top teams, and could impact matchups and home ice for the first round.
No thanks: Jets at Flames is an all-Canadian matchup, which means both teams are terrible.
Entertainment quotient: 5/10 — Not bad, though it’s weird there are only seven games, given it’s the season’s final weekend. They’re probably just saving up to have everyone play on Saturday night. Can’t wait!
Saturday, April 12: Nine games
The can’t-miss matchup: Ducks at Kings — If Anaheim hasn’t wrapped up first place in the Pacific yet, this could be a case where the Ducks have to beat the Kings to avoid a first-round matchup with L.A. And if the division has been locked up, this will be a late-season meeting between two teams that hate each other. Either way, sign me up.
Other good options: Any game between Philadelphia and Pittsburgh is worth watching. The Rangers and Habs are both strong playoff teams. San Jose’s visit to Phoenix could help determine the West’s playoff seedings. And the Maple Leafs close out their season with a visit to Ottawa, giving them one last chance to be booed by a home crowd.
No thanks: The Sabres visit Boston, a team that will have nothing to play for and will probably ice a roster of AHL call-ups and random guys found on the street. So, let’s pencil the Bruins in for an 8-1 win.
Entertainment quotient: 7/10 — There are some fun matchups here. But would it kill the league to give us more than nine games on the season’s final Saturday?
Sunday, April 13: 10 games
The can’t-miss matchup: Dallas at Phoenix — This one is shaping up to be a de facto playoff game, with the winner earning the West’s final wild-card spot and the loser going home.
Other good options: The Avs face the Ducks in a battle of 100-plus-point powerhouses. The Flames and Canucks might at least feature a coach fight or two. And the Red Wings visit St. Louis in a game that could make or break their playoff push — and you know that Blues fans would love nothing more than to be the team that finally ends the playoff streak of their longtime rivals.
No thanks: Just for fun, they should intersperse coverage of the Islanders/Sabres game with footage of Thomas Vanek smiling while wearing a Montreal uniform.
Entertainment quotient: 8/10 — That’s probably being generous, but screw it. The season is over. It’s playoff time.

Hockeytown Endures
Few cities have as rich a cultural and sporting history as Detroit. From the ’80s Pistons to Bob Seger, Eminem to Miguel Cabrera, the Motor City is a rich tapestry of compelling figures, unbelievable moments, and uniquely American ingenuity.
On April 17, ESPN will premiere 30 for 30: Bad Boys, a documentary about those unforgettable Pistons teams. To celebrate, Grantland will devote an entire week, from April 11 through April 18, to the various stories of this wholly original place.
♦♦♦
So, what were you up to in 1991? Me, I can’t be entirely sure, but it definitely involved some combination of gymnastics, slap bracelets, and watching Nickelodeon-produced PSAs about acid rain. If it helps trigger your memory: The Soviet Union was dissolving that year, Super Nintendo came to the U.S., and the ribbon was being cut on California’s first Starbucks. The Silence of the Lambs and “Losing My Religion” were released, and Emma Roberts and Mike Trout were born. And the Detroit Red Wings, one season removed from a pitiful fifth-place division finish, ended their year in the Norris’s third spot to qualify for the NHL playoffs.
That was 23 years ago, and Detroit hasn’t missed the postseason since.
Think about that: There are people, an entire generation of people, who have been born and have grown up and had their hearts broken by high school soul mates and gone off to college and graduated and are now tentatively finding their way through the world. And these people have never known what it’s like to see their favorite hockey team miss the playoffs.
The Red Wings’ 23 years constitutes the longest-running active playoff streak in any of the four major professional sports, and the fifth-longest in hockey history.44 Nine of 30 NHL teams, including the Predators, Lightning, and Senators, didn’t exist in their current iterations in that spring of 1991. The San Jose Sharks first dropped the puck on their franchise later that fall; the team is now second to Detroit in active consecutive NHL postseason appearances. (The Sharks would have to keep making it for another 13 years to even things up.) A couple of players on this year’s Red Wings roster hadn’t even been born then, and so of course it was one of them, 22-year-old Riley Sheahan, who scored the goal last week that guaranteed the streak would live on for at least one more year.
Detroit was playing the Pittsburgh Penguins on that night last week, the Red Wings’ fifth game in a week that began with a big home win over the arguably-best-in-league Boston Bruins. Down 3-2 with a little more than a minute left in the third period against Pittsburgh, the Red Wings iced a line of Sheahan, Tomas Tatar, and Tomas Jurco, three young players with minimal NHL experience who have unexpectedly combined to be one of Detroit’s most consistent lines this season. The trio, out against competition like Sidney Crosby and Kris Letang, responded by netting the game-tying goal. The Penguins would ultimately win in a shootout, but it didn’t really matter: Detroit needed only one point to clinch its 23rd straight playoff berth, and earned it with the regulation tie.
“Maybe people aren’t paying attention to us because of the youth,” Sheahan said that night, “but we build off it, and we have fun with it.”
♦♦♦

Gregory Shamus/NHLI via Getty Images
About a week earlier, Sheahan was at his stall in the Red Wings’ dressing room after practice for an interview with some TV guys from the local Fox Sports affiliate. He sat on a low stool covered in tan leather that looked like it might date back quite a few presidential administrations. His teammate Kyle Quincey hovered behind one of the cameramen, making silly faces at Sheahan, trying to get him to break. (Sheahan mostly held strong.) Across the way, rookie Luke Glendening sauntered past Gustav Nyquist, whose performance in the second half of the season elevated him into a leaguewide sensation, and casually no-look-knocked the Reebok hat right off Nyquist’s head. Goalies Jimmy Howard and Jonas Gustavsson sat in their alcoves bantering as they loosened their heavy equipment.
For an organization often spoken about in hushed tones as the most professional in the league, the vibe during this late-season playoff push was markedly chill.
Players are often loath to admit they keep track of the ever-shifting postseason odds and points standings — We just need to worry about our own games is what they always repeat — but being in the Red Wings dressing room, it’s easy to see how that can’t really be true. In an adjacent hallway, there are two enormous dry-erase boards, both marked off into grids and impossible to ignore. One of them lists every NHL team’s ongoing stats — power-play percentage, goals, shot percentage, that kind of stuff — while the other features the NHL standings. It’s all written in black, except for the Detroit line items, which are bright red.
At one point, in mid-March, the hand-scrawled news must have seemed dire. Following a 4-1 loss to the Blackhawks on March 16, Sports Club Stats calculated that the Wings had just a 35 percent chance of making the playoffs, their lowest level all year. It was certainly understandable. Throughout the season, the Red Wings had been crippled by injuries to the core of the team, and since the Olympic break, things had gotten even worse.
Pavel Datsyuk, a perennial Selke Trophy candidate for his two-way play and a guy with stickhandling so deft, he’s known (even by Siri!) as “The Magic Man,” was out indefinitely with a knee injury that had nagged him before and throughout the Games. Team captain Henrik Zetterberg came back from Sochi hurt, too, with a herniated disc so painful that he couldn’t so much as board a plane out of Russia for days. The list of injuries also included Johan Franzen and Daniel Cleary, and by this point the Red Wings were basically populated by players from their minor league affiliate, the Grand Rapids Griffins. On trade deadline day, the team made an eleventh-hour transaction to acquire David Legwand from the Predators when it realized it had been whittled down to having basically zero centers.
But as other playoff-bubble hopefuls like the Toronto Maple Leafs and the Washington Capitals imploded spectacularly, the Red Wings pressed on, slowly racking up wins. It helped, forward Justin Abdelkader later said, that last year the team found itself in a remarkably similar position, fighting for one of the last couple playoff spots all the way up until the final day of the season.
“The last 20 games were playoff-type,” he said. “Each and every one.”
♦♦♦

HBO’s ’24/7′
Despite barely getting in, last year’s Wings won their first-round matchup against the Ducks in seven games and then very nearly unseated eventual Stanley Cup champion Chicago, leading the series 3-1 before falling in overtime of Game 7. All you have to do is get in, as they say. The players don’t offer up too much about the streak — as with the standings, they never want to let on that their vision is anything but laser-focused on the next 60 minutes — but several of their rookies admitted the specter of it was, in its way, highly motivating: They sure didn’t want to be the guys responsible for ending the two-decades-plus unblemished record of success.
One day while I was in Detroit, I went to the Henry Ford Museum in nearby Dearborn, which included a hypnotic tour of the factory where F-150s are still produced. The propaganda video about the museum’s namesake that played before we were allowed in to view the production line was hagiographic, sure, but it was as stirring as it was designed to be, a reminder of the grandeur and grit of early American manufacturing and industry. It was also bittersweet, depicting the rise of a middle class that has been significantly depleted since.
The rest of the museum was a paean to the constant, unyielding power of innovation and advancement, and not just in regard to cars, but also planes, electricity, consumer electronics (Internet in a Box!), and even chairs. Walking around, it was hard not to think about how this theme of forward momentum was in many ways at odds with the museum’s surrounding environs.
Detroit had a population of more than 1 million back in 1991; it’s since dwindled to just north of 700,000. No matter how optimistic one is about the potential for urban renewal, the deterioration of Detroit is evident everywhere. The fancy Westin hotel where visiting NHL teams routinely stay is directly across the street from a vacant brick building with an old closed-down Quiznos that looks borderline burned-out. Around the city are various signs informing you that the stoplights at that particular intersection are under review for removal; the birthplace of automobiles is now adjusting for lighter traffic.
♦♦♦

Gregory Shamus/Getty Images
Going to a game at Joe Louis Arena feels like emerging from a time machine. The ice-level hallways are covered with old names and accolades, each painted in stern red block letters on the whitewashed brick walls. The banner ads that dot the stands are sponsored by the kind of local area businesses you usually see in minor league rinks. During intermission, there is scarcely any arena entertainment. The light sconces outside the private suites are decorated with art deco moldings, each one like its own little Chrysler Building. Reporters who cover the games perch on what are basically bar stools. (One writer told me the press box was originally designed as a bar, until the builders realized they’d forgotten to account for the media.)
But the Wings won’t be playing in Joe Louis for much longer; in 2016, a controversial new $650 million arena is scheduled to open that will be financed, in part, by $284.5 million of the bankrupt city’s public funds.45 The building will go up not far from Comerica Park, which was built in 2000 and where I spent a beautiful day watching baseball after roughly 700 folks implored me to do so. “Opening Day is a holiday in Detroit!” was the familiar refrain, and I couldn’t help but think that the true annual celebration, after all these years, really ought to be one honoring hockey.
♦♦♦

Dave Reginek/NHLI via Getty Images
These aren’t your childhood Red Wings. The late-’90s iterations of the franchise were in some ways as close to a dynasty as it got in the post–Gretzky’s Oilers era. (Detroit went to the Stanley Cup final four times in eight seasons, winning three times, and that’s not even counting the 2008 Cup it also earned.) The team’s dogged ingenuity in wooing Russian superstars as the Berlin Wall was beginning to wobble endeared Detroit to an international audience. (Nearly all the Russians I’ve unofficially polled during my travels name the Wings as their favorite NHL franchise, a relic from those heady days.) “Hockeytown” became a household name, and octopi routinely flew triumphantly through the air.
The team had a borderline apocryphal history of success in the NHL draft: It nabbed Nicklas Lidstrom in the third round in 1989 with the help of a wee bit of subterfuge; took Datsyuk 171st overall in 1998; and snagged Zetterberg in the seventh round a year later. Better to be lucky than good, you could argue, but the Wings have always seemed to be both.
There’s a verifiable diaspora of Red Wings who have fanned out through the hockey world in their retirement: Steve Yzerman just re-upped his contract as GM of the Tampa Bay Lightning; Brendan Shanahan is the newly named president of the Leafs (best of luck, buddy); Sergei Fedorov runs Dynamo Moscow in the KHL; and Kirk Maltby and Kris Draper are Red Wings scouts, just to name a few.

Steve Babineau/NHLI via Getty Images
Glendening is one of several players on the roster who grew up in Michigan. He recalls listening to Red Wings games on the radio in bed when they dragged on too late for him to be allowed to watch on TV, and says he idolized Yzerman. Danny DeKeyser wore no. 5 in college hockey in homage to Lidstrom. Legwand, who is from Grosse Pointe, loved Yzerman, too — what good local kid wouldn’t? — and recalls attending games at Joe Louis Arena.
This year’s team, though, hasn’t dominated like those guys once did — more accurate to say it has endured. Much is made of the success of Detroit’s AHL team, the Griffins, who won last year’s Calder Cup and upon whom the Red Wings have had to rely heavily this year for roster players. It’s true the two teams are remarkably in sync, playing with the same systems and having a proven track record of players making strong NHL adjustments. But it’s not necessarily that the Wings do a better job of developing players than other teams — it’s that the franchise has long had the luxury of depth and thus plenty of time. Many players spend a token year or two in the minor leagues and are often called up a little too early by their NHL teams, but Detroit has sometimes had a different problem. Coach Mike Babcock liked what he saw from a guy like Glendening in training camp, but there was no room to really keep him. That is, until the injuries piled up.
Babcock is one of those coaches whose consistent results in such a well-oiled organization have perversely worked against him in annual Jack Adams voting, which typically tends to reward coaches presiding over once-volatile programs on the upswing. But this season might be one in which his talents are more formally recognized; while his Olympic coaching performance and his part in Red Wings history aren’t technically meant to enter into the voting decision, he kind of deserves something like a lifetime achievement award.
One of the many injured players for the Wings this season, Datsyuk, was making his long-awaited return when I was in Detroit. “The whole thing is to understand it’s not about Pavel one bit,” said Babcock, who had just returned from his routine post-practice jog around Joe Louis. “It’s about the Red Wings, and the guys that are playing, and we just got to keep grinding.”
The guys that were playing included Nyquist, who leads the league in goals since January 20 thanks to an unsustainably high but undeniably entertaining shot percentage spike, as well as Sheahan and Jurco and Tatar and other young players like Brendan Smith, DeKeyser, and Glendening. Ask them what they were doing in 1991, and they have no answers, because they were too young to be truly sentient. After the playoff-clinching Pittsburgh game, Babcock paid tribute to the multitude of rookies (or, in the case of Nyquist, close to it) on his squad. Even with Datsyuk back, and with Daniel Alfredsson in and out of the lineup, he’ll continue to rely on them as Detroit chases the Cup. (Again.)
“They came here and they took jobs,” he said. “They’re not going anywhere. They’re real good players that keep getting better and they’ll be part of us for a long time … We’re a way quicker, harder team, we’re a more physical team.
“And we can’t be backed off,” he continued. “I like us.”
Illustration by Gluekit.

The Lowe Post Podcast: Zach Lowe and Spurs GM R.C. Buford
Zach talks to Spurs GM R.C. Buford about conference realignment, the team’s culture, and whether he’d ever leave.
Listen to the podcast here. Subscribe to the B.S. Report on iTunes, and check out our podcasts page.
To subscribe to Grantland’s podcast YouTube channel, click here.

6 high-priced Android apps worth your hard-earned money
Let’s be honest, when it comes to buying apps we can all be a bit stingy with our cash. Most of us have no problem shelling out $200 for the latest Samsung device, but dropping a couple of bucks on some app seems so much more difficult. It’s a very strange situation. Through the years most people have decided that any app that costs over $1.99 is “expensive.” We really need to get over this mentality.
Most people have no problem spending a few bucks on a Big Mac that will keep them satisfied for a couple of hours. Why is it so hard to buy $5 app that you will use every day of your life? Not to mention the money you spend on apps is supporting hard-working developers. We think there are plenty of apps out there that are worthy of your hard-earned cash. Here are 5 “high” priced apps and 1 cheapy for your consideration.
Link Bubble – $4.99
Link Bubble is a completely new way to browse the web on your Android device. When you click on a link the webpage begins to load in the background, allowing you to continue using the app you are in. Once the page is loaded it pops up on top of the current app. You never have to leave the app you are using or wait for pages to load. $5 may seem a bit steep, but when you consider how many times you open links it’s well worth it.
Plex – $4.99
If you know anyone that uses Plex they have probably already told you how great it is. This service can be easily described by their tagline: “One window into all your personal media. No matter where you are.” Upload all of your media to the Plex server and it can be accessed anywhere you can download the app. Photos can be automatically uploaded, and videos are displayed with beautiful movie posters. Plex even works with Chromecast. The $5 will give you access to all of this on your phone or tablet.
Safe In Cloud – $4.99
If you use the same password on every site, or let browsers remember your login info, you are at risk of getting hacked. Having a unique login and password for every site can be incredibly difficult to remember. Safe In Cloud is a password management system. that helps you keep track of it all. You can sync your passwords to Google Drive, Dropbox, or OneDrive. The $5 will get you the Android app, plus the Chrome and Firefox extension for free. Privacy is nothing to skimp out on.
Pushover – $4.99
We all gets tons of notifications, but rarely about the things that should really be notifying us. Pushover can make notifications work for you. Want to know when your Fitbit battery is low? When a completely-legal-in-every-way torrent finishes downloading? An eBay item changes price? With Pushover you can get notifications for all of these things, and many more. Dozens of apps, services, and things can be configured to work with Pushover. For $5 you will be notified of anything and everything you can imagine.
ROM Manager – $5.99
If you are a frequent user of custom ROMs you have probably used the free version of ROM Manager at some time. This incredibly useful app comes pre-installed with some ROMs. The premium version adds a few features that are very important for hardcore ROM users. You can download incremental update zips to save on data, get access to premium ROMs, and receive notifications for when your ROM is updated. If you’ve ever hassled with ROMs this is an easy $6 to spend.
MarkAsRead – $1.21
Our cheapy pick is a new app that solves a small but annoying problem. Gmail’s current notifications let you archive and reply to emails directly from the notification. Unfortunately there is no way to mark an email as “read” from there. MarkAsRead replaces the stock Gmail notification with an almost identical one, except now there is a “read” button. No more seeing new emails twice. For just $1.21 this will make your life easier.
Those are some of the apps we think are worth the price tag, but what about you? What apps do you consider to be good enough to buy? Let us know in the comments below!
Mobile Roar Podcast 39: Amazon Fire TV & Cortana [Poll]
Amazon has finally made their move to take over the living room, but is Fire TV any better than existing set-top boxes? Do people want to play Android games on their TV We also talk about Microsoft conforming to the competition, and releasing a feature with the coolest name ever. Lastly, we share our best and worst April Fool’s Day jokes and recommend some apps. Thanks for watching/listening!
Big News
- Amazon Fire TV
- Windows Phone 8.1 official
Best Phones/Tablets as of April
Quick Hits
- WWDC begins June 2nd, developer ticket lottery starts this week
- Apple/Samsung trial begins
- Google looking to become a MVNO on Sprint/Verizon network
- Google apps are getting redesigned (Gmail leak)
- Google readies standalone camera app for a Google Play
- Google bans erotica from the Play Store
- Vine testing camera roll upload
Best and Worst April Fool’s Jokes
App Picks
- Kevin: MLB At Bat
- Joe: Star Wars Assault Team
- Chris: Hover Browser
Follow Us
- Joe Fedewa (@tallshmo)
- Chris Chavez (@GamerCore)
- Kevin Krause (@youdontknowkev)
![]() |
![]() |
![]() |
![]() |
![]() |